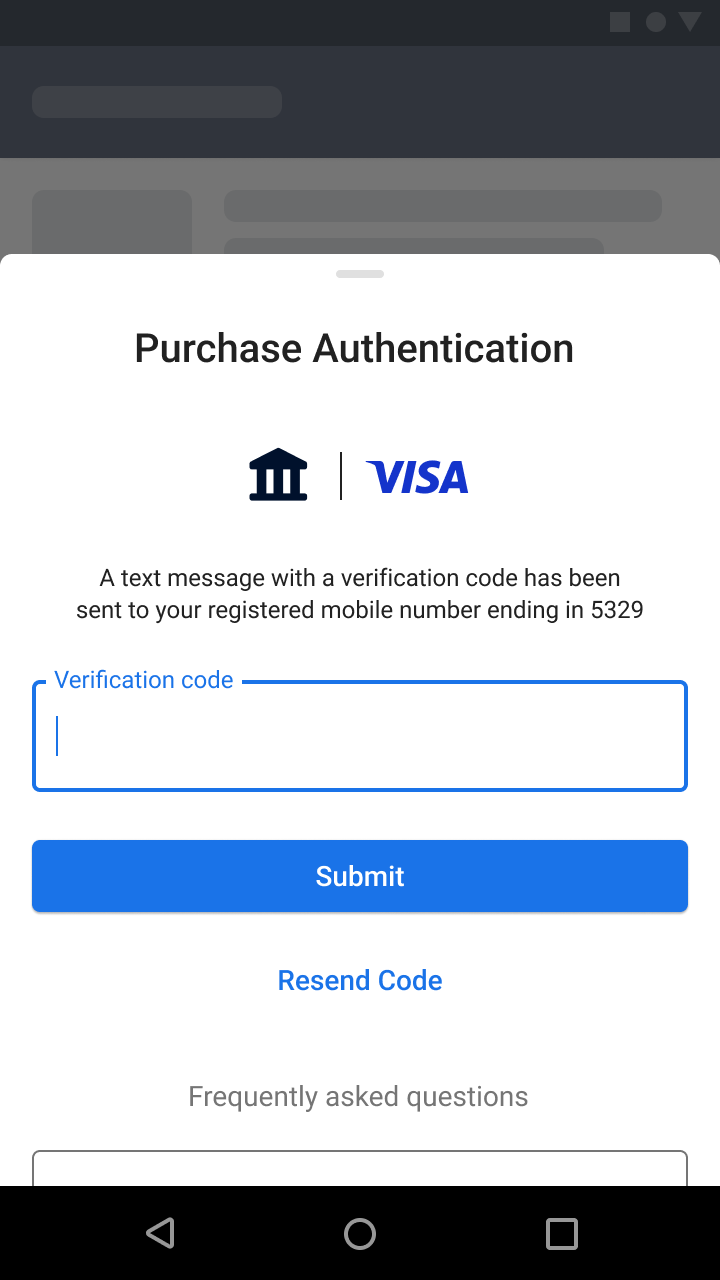
Android Drop-in
Provide a better experience by performing native 3D Secure 2 authentication in your Android app.
Use Drop-in to render the available cards in your payment form and securely collect card information, so sensitive data does not reach your server.
Drop-in handles the 3D Secure 2 frictionless and challenge flows, including the data exchange between your front end and the issuer's Access Control Server (ACS).
Other 3D Secure flows
With a native Android Drop-in 3D Secure 2 integration, you can also support:
Start integrating with Android Drop-in
Choose your versionThis page explains how to implement native 3D Secure 2 authentication with your existing Android Drop-in integration.
Requirements
Before you begin, take into account the following requirements, limitations, and preparations.
Requirement | Description |
---|---|
Integration type | Make sure you have an advanced flow Android Drop-in integration. |
Webhooks | Subscribe to Standard webhooks. |
Setup steps | Before you begin:
|
How it works
For a Drop-in integration, you must implement the following parts:
The parts of your integration work together to complete the payment flow:
- From your server, submit a request to get a list of payment methods available to the shopper.
- Configure and launch Drop-in to collect the shopper's details.
- From your server, make a payment request with data that you receive from Drop-in.
- Handle additional client-side actions, if required.
- From your server, send additional payment details with data you receive from Drop-in, if required.
- Get the payment outcome to inform the shopper and update your order management system.
If you are integrating these parts separately, you can start at the corresponding part of this integration guide:
Collect additional parameters in your payment form
For higher authentication rates, we strongly recommend that you collect the shopper's billing address and email address. Send these parameters to your server when making a payment, because they are required by the card schemes.
Optional configuration
When you configure the Drop-in, you can include the following functions:
Configuration function | Description | Parameter |
---|---|---|
setThreeDSRequestorAppURL |
Strongly recommended to improve conversion rates. Set this to an Android App link to call your app after an out-of-band (OOB) authentication occurs. When set, your app must also handle this Android App link. We do not recommend to use custom links because they can be defined and used by other apps. This causes errors if two apps on the same device have the same custom link. |
threeDSRequestorAppURL : String.Default: null. |
setUiCustomization |
Customization for the 3D Secure 2 authentication UI. | A
UiCustomization
object. |
For example:
// Create a configuration object. val checkoutConfiguration = CheckoutConfiguration( environment = environment, clientKey = clientKey, ) { // Configure 3D Secure 2. adyen3DS2 { setThreeDSRequestorAppURL("https://your.app.com/adyen3ds2") // Strongly recommended. } }
After configuring Drop-in, continue to launch and show Drop-in and make the payment request.
Make a payment
When you make a payment request, include additional parameters for 3D Secure 2 in the /payments request from your server:
Parameter name | Required | Description |
---|---|---|
paymentMethod | ![]() |
If submitting raw card data, send the required payment method parameters. |
paymentMethod.holderName |
Required for Visa | The cardholder's name. |
paymentMethod.threeDS2SdkVersion | ![]() |
Required to trigger in-app native. See how to get the SDK version. |
browserInfo | ![]() |
The shopper's browser information. Include the following fields to handle cases where the payment is routed to 3D Secure 2 redirect.
|
channel | ![]() |
Set to Android. |
authenticationData.threeDSRequestData.nativeThreeDS | ![]() |
Set to preferred. Indicates that your payment page can handle 3D Secure 2 transactions natively. |
returnUrl | ![]() |
The URL that the shopper will be redirected to after they complete the payment. We strongly recommend to redirect the shopper to your app by configuring a threeDSRequestorAppURL , because this improves conversion. |
billingAddress | Strongly recommended | The cardholder's billing address. |
shopperEmail | Required for Visa | The cardholder's email address. shopperEmail or a phone number is required for Visa. |
threeDS2requestData.homePhone ,threeDS2RequestData.workPhone orthreeDS2RequestData.mobilePhone |
Required for Visa | A phone number for the shopper. shopperEmail or a phone number is required for Visa. |
To increase the likelihood of achieving a frictionless flow and higher authorisation rates, we recommend that you send additional parameters if you have the data available. Do not send placeholder data in the live environment.
For channel
Android, we recommend including these additional parameters: billingAddress
, shopperEmail
, and shopperIP
.
Get the SDK version
Use the ThreeDS2Service.INSTANCE.getSDKVersion()
function to determine your 3D Secure 2 sdkVersion
. You can also get the sdkVersion
from Github. We recommend not to hardcode the SDK version.
curl https://checkout-test.adyen.com/checkout/v69/payments \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d '{ "amount":{ "currency":"EUR", "value":1000 }, "reference":"YOUR_ORDER_NUMBER", "shopperReference":"YOUR_UNIQUE_SHOPPER_ID", "paymentMethod": STATE_DATApaymentMethod field of an object passed from the client app. Return the fields as they are, sdkVersion is required to trigger native., "authenticationData": { "threeDSRequestData": { "nativeThreeDS": "preferred" } }, "billingAddressstate.data.billingAddress from onSubmit": { "street": "Infinite Loop", "houseNumberOrName": "1", "postalCode": "1011DJ", "city": "Amsterdam", "country": "NL" }, "browserInfoRequired for 3D Secure 1: { "userAgent":"Mozilla/5.0 (Linux; Android 6.0.1; Nexus 6P Build/MMB29P) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/47.0.2526.83 Mobile Safari/537.36", "acceptHeader":"text\/html,application\/xhtml+xml,application\/xml;q=0.9,image\/webp,image\/apng,*\/*;q=0.8" }, "shopperEmail":"s.hopper@example.com", "channel":"Android", "returnUrl":"adyencheckout://your.package.name", "merchantAccount":"YOUR_MERCHANT_ACCOUNT" }'
Your next steps depend on whether the /payments response contains an action
object, and on the action.type
:
action.type |
Description | Next steps |
---|---|---|
No action object |
The transaction was either exempted or out-of-scope for 3D Secure 2 authentication. | 1. Return DropInServiceResult.Finished , and the resultCode from the /payments response. 2. Use the resultCode to present the payment result to your shopper. |
threeDS2 | The payment qualifies for 3D Secure 2, and will go through the authentication flow. | 1. Return DropInServiceResult.Action and the action object. 2. Submit authentication results. |
redirect | The payment is routed to the 3D Secure 2 redirect flow. |
1. Return DropInServiceResult.Action and the action object. 2. Check the payment result. |
The following example shows a /payments response with action.type
: threeDS2
{ "action":{ "type":"threeDS2", "subtype": "fingerprint", "paymentData":"Ab02b4c0!BQABAgCuZFJrQOjSsl\/zt+...", "paymentMethodType":"scheme", "authorisationToken" : "Ab02b4c0!BQABAgAvrX03p...", "token":"eyJ0aHJlZURTTWV0aG9kTm90aWZpY..." }, "resultCode":"IdentifyShopper", ... }
Submit additional 3D Secure 2 authentication details
When you send additional details, send the 3D Secure 2 authentication data from your server:
-
Drop-in calls the
onAdditionalDetails
method in yourDropInService
class passing, theactionComponentJson
object. Pass the object to your backend server. -
From your server, make a POST /payments/details request, including
actionComponentJson
object:
curl https://checkout-test.adyen.com/checkout/v70/payments/details \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d 'STATE_DATAobject passed from the front end or client app'
- Pass the /payments/details response from your server to your client-side app.
Continue and complete your payment flow.
Present the payment result
Use the resultCode from the /payments or /payments/details response to present the payment result to your shopper. You will also receive the outcome of the payment asynchronously in a webhook.
For card payments, you can receive the following resultCode
values:
resultCode | Description | Action to take |
---|---|---|
Authorised | The payment was successful. | Inform the shopper that the payment has been successful. If you are using manual capture, you also need to capture the payment. |
Cancelled | The shopper cancelled the payment. | Ask the shopper if they want to continue with the order, or ask them to select a different payment method. |
Error | There was an error when the payment was being processed. For more information, check the
refusalReason
field. |
Inform the shopper that there was an error processing their payment. |
Refused | The payment was refused. For more information, check the
refusalReason
field. |
Ask the shopper to try the payment again using a different payment method. |
Handle your Android App Link
If you have configured the setThreeDSRequestorAppURL
when you configured Drop-in, you have to handle this Android App link.
-
Add the following to your
AndroidManifest.xml
, specifying your Android App Link as yourandroid:host
:Copy to your app Manifest fileExpand viewCopy link to code blockCopy code<activity android:name="com.adyen.threeds2.internal.ui.activity.ChallengeActivity" android:exported="true" tools:node="merge"> <intent-filter android:autoVerify="true" tools:targetApi="m"> <action android:name="android.intent.action.VIEW" /> <category android:name="android.intent.category.DEFAULT" /> <category android:name="android.intent.category.BROWSABLE" /> <data android:host="{your.app.com}" android:pathPrefix="/adyen3ds2" android:scheme="https" /> </intent-filter> </activity>
Testing
Use our test card numbers to test how your integration handles different 3D Secure authentication scenarios.
Troubleshooting
If native 3D Secure 2 is not triggered, check that:
- Your Component version is 4.9.1 or later.
paymentMethod.threeDS2SdkVersion
is populated and sent in payment request.channel
is set to Android.authenticationData.threeDSRequestData.nativeThreeDS
is set to preferred if you use Checkout API v69 or later ORadditionalData.allow3DS2
is set to true if you use Checkout API v68 or earlier.
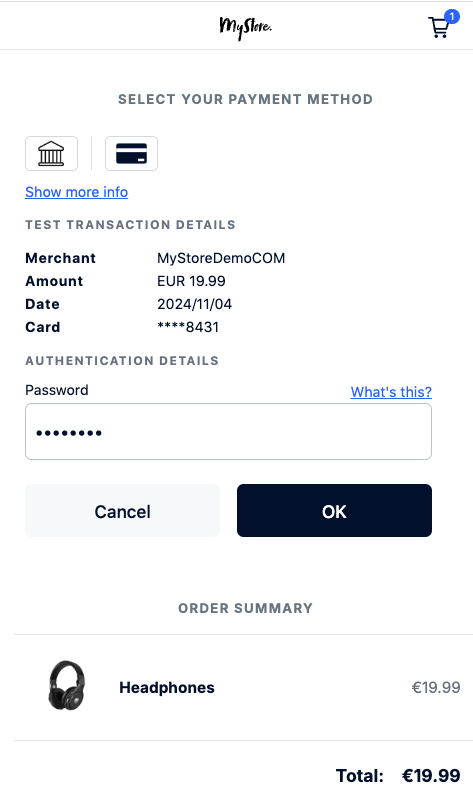
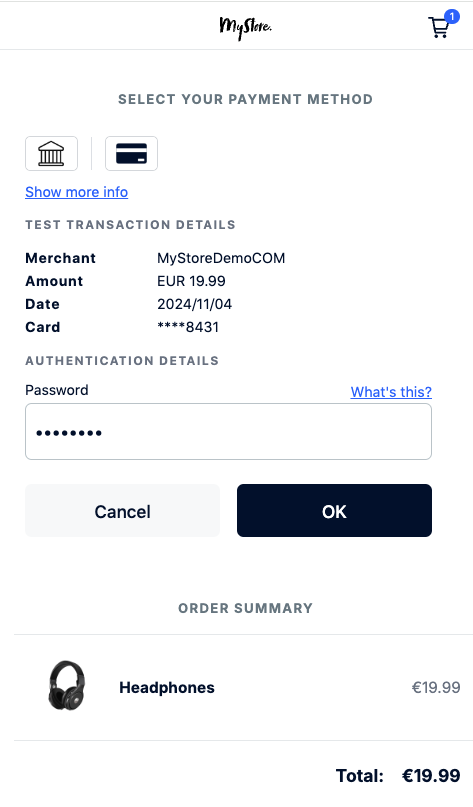
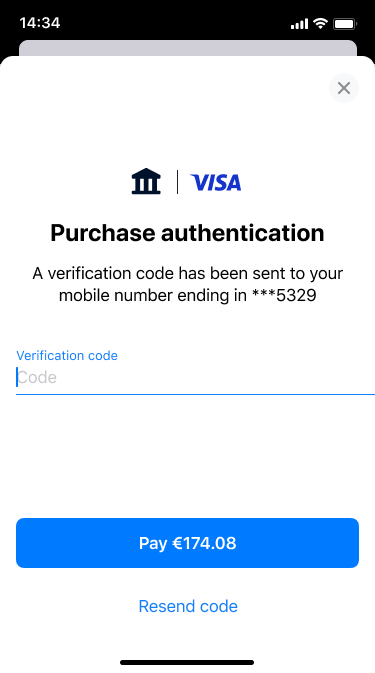
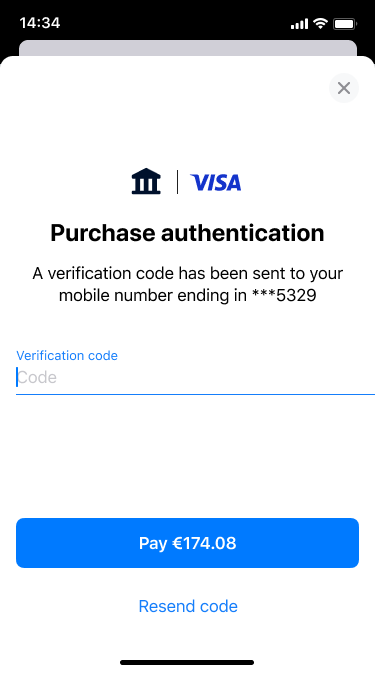
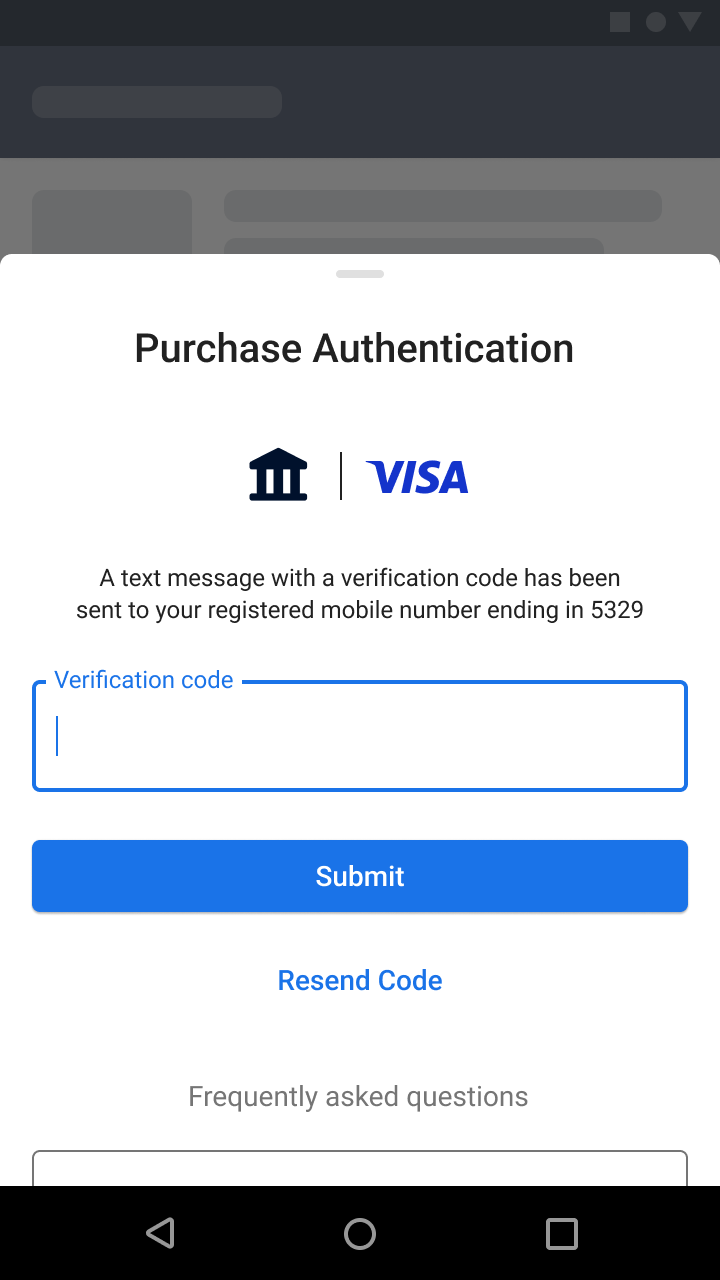
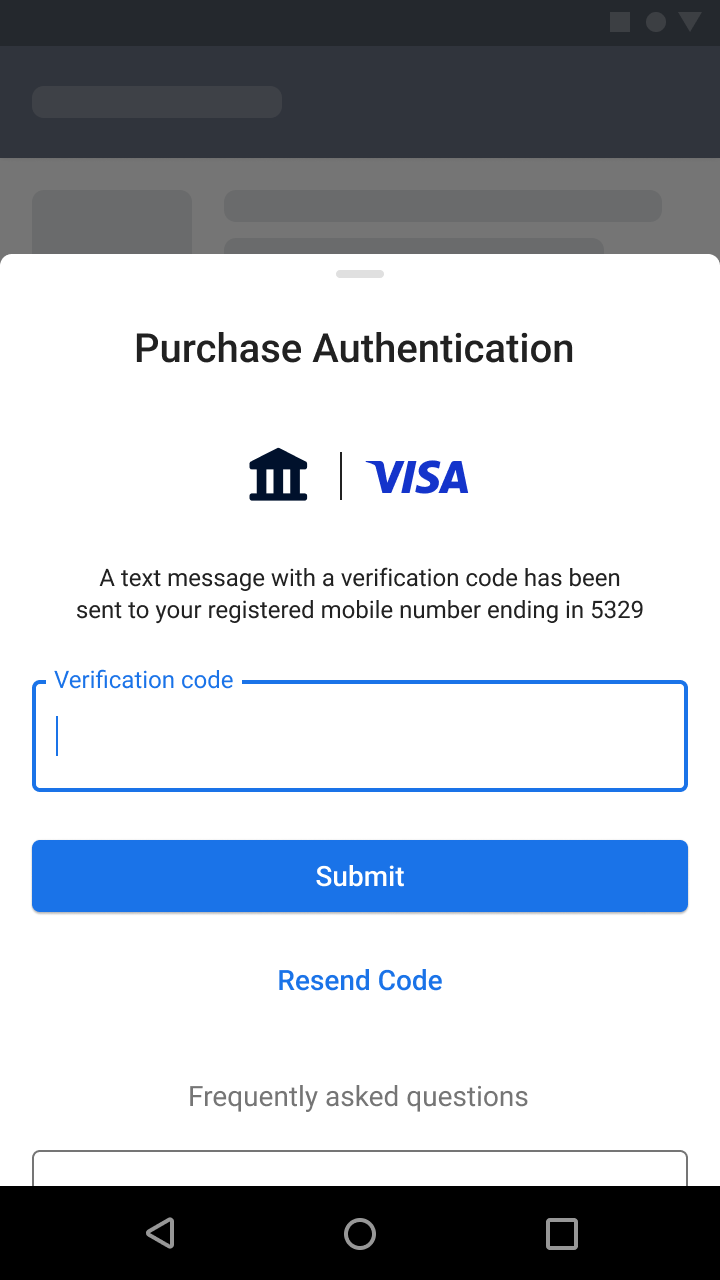