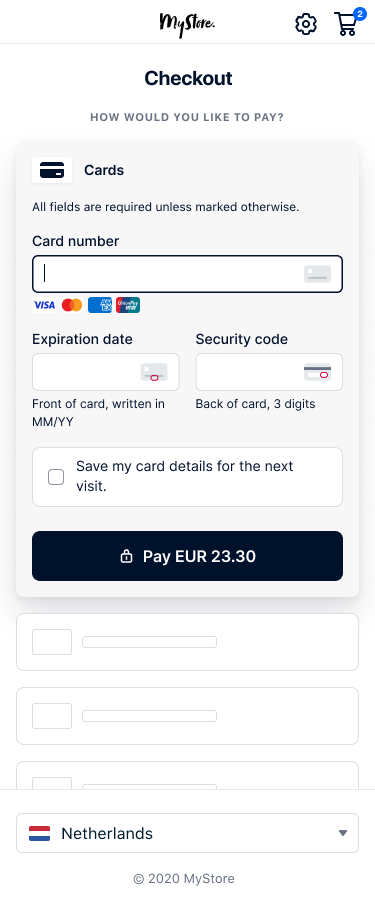
Web Components
Render individual payment methods anywhere on your website.
Supported payment methods
Cards, buy now pay later, wallets, and many more.
See all supported payment methods
Features
- Low development time to integrate each payment method component
- UI styling customization for each payment method
- Flexibility to add payment method components with configuration for each
- 3D Secure 2 support using the 3D Secure 2 Component
Demo
Start integrating with Web API only
Choose your versionUse our APIs and build your own payment form if you want full control over the look and feel of your checkout page. If you'd rather not build your own payment form, use one of our pre-built UI options.
With our API-only solution, you have two options for accepting card payments:
- Use our custom card integration with encryption to securely encrypt card details. This helps you ensure PCI compliance, because you are only required to submit SAQ A.
- Collect and pass raw card data. This requires you to assess your PCI compliance according to SAQ D, the most extensive form of self-certification.
Requirements
If you want to accept payments using raw card data, confirm with your Adyen Account Manager that you are eligible to use this option.
Before you begin to integrate, make sure you have followed the Get started with Adyen guide to:
- Get an overview of the steps needed to accept live payments.
- Create your test account.
After you have created your test account:
- Get your API key.
- Get your client key.
- Set up webhooks to know the payment outcome.
To make sure that your 3D Secure integration works on Chrome, your cookies need to have the SameSite attribute. For more information, refer to Chrome SameSite Cookie policy.
How it works
For a integration, you must implement the following parts:
Integration steps
The parts of your integration work together to handle the payment flow:
- From your server, make an API request to get a list of payment methods available to the shopper.
- Show your UI that includes the payment form to collect the shopper's payment details.
- From your server, make a payment request with the data that you have collected from the shopper.
- For some payment methods, your client handles the additional action that your shopper must perform. For example, redirect your shopper to another website or show a QR code that the shopper uses to complete the payment.
- From your server, send additional payment details.
- Get the payment outcome.
If you are integrating these parts separately, you can start at the corresponding part of this integration guide:
Install an API library
We provide server-side API libraries for several programming languages, available through common package managers, like Gradle and npm, for easier installation and version management. Our API libraries will save you development time, because they:
- Use an API version that is up to date.
- Have generated models to help you construct requests.
- Send the request to Adyen using their built-in HTTP client, so you do not have to create your own.
Try our example integration
Requirements
- Java 11 or later.
Installation
You can use Maven, adding this dependency to your project's POM.
<dependency> <groupId>com.adyen</groupId> <artifactId>adyen-java-api-library</artifactId> <version>LATEST_VERSION</version> </dependency>
You can find the latest version on GitHub. Alternatively, you can download the release on GitHub.
Setting up the client
Create a singleton resource that you use for the API requests to Adyen:
// Import the required classes. package com.adyen.service; import com.adyen.Client; import com.adyen.service.checkout.PaymentsApi; import com.adyen.model.checkout.Amount; import com.adyen.enums.Environment; import com.adyen.service.exception.ApiException; import java.io.IOException; public class Snippet { public Snippet() throws IOException, ApiException { // Set up the client and service. Client client = new Client("ADYEN_API_KEY", Environment.TEST); } }
Get available payment methods
When your shopper is ready to pay, get a list of the available payment methods based on their country, device, and the payment amount.
-
From your server, make a POST /paymentMethods request, specifying:
Parameter name Required Description merchantAccount
Your merchant account name. amount
The currency
andvalue
of the payment, in minor units. This is used to filter the list of available payment methods to your shopper.channel
The platform of the shopper's device: Web, iOS, or Android. This is used to filter the list of available payment methods to your shopper. countryCode
The shopper's country/rgion. This is used to filter the list of available payment methods to your shopper.
Format: the two-letter ISO-3166-1 alpha-2 country code. Exception: QZ (Kosovo).shopperLocale
Language and country code. This is used to translate the payment methods names in the response. By default, the shopperlocale
is set to en-US.The following example shows how to get the available payment methods for a shopper in the Netherlands, for a payment of EUR 10:
Expand viewCopy link to code blockCopy codecurl https://checkout-test.adyen.com/checkout/v70/paymentMethods \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d '{ "merchantAccount": "ADYEN_MERCHANT_ACCOUNT", "countryCode": "NL", "amount": { "currency": "EUR", "value": 1000 }, "channel": "Android", "shopperLocale": "nl-NL" }'
The response includes the list of available paymentMethods
, ordered by popularity in the shopper's country. For each payment method, the response contains:
name
: Name of the payment method, which you can display to your shopper in your payment form.-
type
: Unique payment method code. You'll need to pass this when making a payment request./paymentMethods responseExpand viewCopy link to code blockCopy code{ "paymentMethods":[ { "name": "Cards", "type": "scheme" }, { "name":"SEPA Direct Debit", "type":"sepadirectdebit" }, { ... } ] }
- Provide the list of payment methods and the required input fields for each payment method to your client app.
Collect shopper details
For card payments, you must collect your shopper's card payment details based on your PCI compliance.
Collect your shopper's payment details based on the fields required by each payment method. Check the payment method pages to see which fields are required for each payment method.
We provide payment method and issuer logos which you can use in your payment form. For more information, refer to Downloading logos.
After you have collected your shopper's payment details, pass the data and the payment method type
to your server.
For example:
{ "type": "sepadirectdebit", "sepa.ownerName": "A Schneider", "sepa.ibanNumber": "NL13TEST0123456789" }
Collecting your shopper's card details
If the shopper selects to pay with a Card payment method, you have the following options for collecting card details:
- Use our custom card integration with encryption to encrypt your shopper's card data.
- Collect your shopper's raw card data, if you have confirmed with us that you are eligible to use this option.
If you are building your own UI, we provide payment method and issuing bank logos that you can use on your checkout page. The images are available in PNG format with different sizes and screen resolutions and in SVG format.
If you cannot find a payment method or issuer logo, contact our Support Team.
Payment method logos
Download the images from the links below, specifying:
-
img-size
: Specify the size for PNG format. Use the following values:- small: Image size 40x26 pixels
- medium: Image size 77x50 pixels
- large: Image size 154 x 100 pixels
-
suffix
: Specify the image density for PNG format. If not specified, the images will have the same size as theimg-size
. Append any of the following values:@2x
@3x
-ldpi
-hdpi
-xhdpi
-xxhdpi
-xxxhdpi
-
pm-type
: ThepaymentMethods.type
returned in the/paymentMethods
response. For example, googlepay or primeiropay_boleto.For cards, the values you should use are specified under
brands
withtype
: scheme. For example,mc
,visa
, andamex
. To get a generic card logo, setpm-type
to card.
Download link for SVG:
https://checkoutshopper-live.adyen.com/checkoutshopper/images/logos/[pm-type].svg
Download link for PNG:
https://checkoutshopper-live.adyen.com/checkoutshopper/images/logos/[img-size]/[pm-type][suffix].png
Examples:
https://checkoutshopper-live.adyen.com/checkoutshopper/images/logos/mc.svg
https://checkoutshopper-live.adyen.com/checkoutshopper/images/logos/large/googlepay@2x.png
Issuing bank logos
Some payment methods such as iDEAL present a list of issuing banks to the shopper.
Download the issuing bank logos from the links below, specifying:
-
img-size
: Specify the size for PNG format. Use the following values:- small: Image size 40x26 pixels
- medium: Image size 77x50 pixels
- large: Image size 154 x 100 pixels
-
suffix
: Specify the image density for PNG format. If not specified, the images will have the same size as theimg-size
. Append any of the following values:@2x
@3x
-ldpi
-hdpi
-xhdpi
-xxhdpi
-xxxhdpi
-
pm-type
: ThepaymentMethods.type
in objects withdetails.key
issuer returned in the/paymentMethods
response. For example, ideal. -
issuerid
: Thedetails.items.id
referring to the issuing bank. For example, 1121 and 1151 for iDEAL.
Download link for SVG:
https://checkoutshopper-live.adyen.com/checkoutshopper/images/logos/[pm-type]/[issuerid].svg
Download link for PNG:
https://checkoutshopper-live.adyen.com/checkoutshopper/images/logos/[img-size]/[pm-type]/[issuerid][suffix].png
Examples:
https://checkoutshopper-live.adyen.com/checkoutshopper/images/logos/ideal/1121.svg
https://checkoutshopper-live.adyen.com/checkoutshopper/images/logos/small/ideal/1151-xxhdpi.png
Make a payment
After the shopper submits their payment details or chooses to pay with a payment method that requires a redirection, you need to make a payment request to Adyen.
From your server, make a /payments request specifying:
Parameter name | Required | Description |
---|---|---|
merchantAccount |
![]() |
Your merchant account name. |
amount |
![]() |
The currency and value of the payment, in minor units. |
reference |
![]() |
Your unique reference for this payment. |
paymentMethod.type |
![]() |
The type of this payment method from the
/paymentMethods
response. |
paymentMethod |
![]() |
The shopper details that you collected in the previous step. |
returnUrl |
![]() |
URL to where the shopper should be taken back to after a redirection. The URL can contain a maximum of 1024 characters. - For Web the URL should include the protocol: http:// or https:// . You can also include your own additional query parameters, for example, shopper ID or order reference number. - For iOS, use the custom URL for your app. For example, my-app:// . For more information on setting custom URL schemes, refer to the Apple Developer documentation. - For Android, use a custom URL handled by an Activity on your app. You can configure it with an intent filter. For example, configure my-app://your.package.name , and then add that to your manifest.xml file. If the URL to return to includes non-ASCII characters, like spaces or special letters, URL encode the value. The URL must not include personally identifiable information (PII), for example name or email address. |
applicationInfo
|
If you are building an Adyen solution for multiple merchants, include some basic identifying information, so that we can offer you better support. For more information, refer to Building Adyen solutions. |
You need to include additional parameters in your payment request to:
- Integrate some payment methods. For more information, refer to our payment method integration guides.
- Make use of our risk management features. For more information, see Required risk fields.
- Use native 3D Secure 2 authentication.
- Tokenize your shopper's payment details or make recurring payments.
The following example shows how to make a credit card payment for EUR 10 using encrypted card data on a website. If you are eligible to process raw card data, you send in different fields.
curl https://checkout-test.adyen.com/checkout/v70/payments \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d '{ "merchantAccount":"ADYEN_MERCHANT_ACCOUNT", "paymentMethod":{ "type": "scheme", "encryptedCardNumber": "test_4111111111111111", "encryptedExpiryMonth": "test_03", "encryptedExpiryYear": "test_2030", "encryptedSecurityCode": "test_737" }, "amount":{ "currency":"EUR", "value":1000 }, "reference":"YOUR_ORDER_NUMBER", "returnUrl":"https://your-company.com/checkout?shopperOrder=12xy.." }'
Your next steps depend on whether the /payments response contains an action
object. Choose your API version:
Description | Next steps | |
---|---|---|
No action object |
No additional steps are needed to complete the payment. | Get the payment outcome. |
With action object |
Additional steps are needed to complete the payment. | 1. Pass the action object to your client app. 2. Perform additional actions. |
The following example shows the /payments response for iDEAL, a redirect payment method.
{ "resultCode": "RedirectShopper", "action": { "paymentMethodType": "ideal", "url": "https://checkoutshopper-test.adyen.com/checkoutshopper/checkoutPaymentRedirect?redirectData=X6Xtf...", "method": "GET", "type": "redirect" } }
Handle the additional action
If your /payments response includes the action
object, what you must do depends on the action.type
:
action.type |
Description | Next steps |
---|---|---|
voucher | The shopper gets a voucher which they will use to complete the payment later. | 1. Show the voucher. 2. Get the payment outcome. |
qrCode | The shopper scans a QR code and is redirected to an app to complete the payment. | 1. Show the QR code. 2. Wait for the notification webhook to know the payment result. |
redirect | The shopper is redirected to another website or app to complete the payment. | Handle the redirect. |
await | The shopper completes the payment asynchronously, for example by using an app. | 1. Inform the shopper that you are waiting for the payment. 2. Submit additional payment details to complete the payment. |
sdk | Applies for payment methods that require an SDK, for example WeChat Pay. The type can sometimes include the payment method name, for example: wechatpaySDK. |
1. Invoke the SDK to trigger the switch to the payment method's app. 2. Submit additional payment details to complete the payment. |
threeDS2 | The payment qualifies for 3D Secure 2 and will go through 3D Secure 2 authentication. | Follow our 3D Secure 2 Components integration guide for your platform. |
Show the voucher or QR code to your shopper
For voucher and QR code actions, you must use the information in the action
object to show something to the shopper.
For the voucher action.type
:
-
Get the voucher data from the
action
object and present this information on your client app.For example, for Indonesian bank transfer payments, you get the following fields in the response:
Parameter Description expiresAt
The voucher expiry date. initialAmount
The amount and currency that the shopper has to pay. merchantName
The name of your shop. instructionsUrl
The link where the shopper can get additional instructions for the payment. Voucher action typeExpand viewCopy link to code blockCopy code{ "resultCode": "PresentToShopper", "action": { "expiresAt": "2021-09-04T19:17:00", "initialAmount": { "currency": "IDR", "value": 10000 }, "instructionsUrl": "https://checkoutshopper-test.adyen.com/checkoutshopper/voucherInstructions.shtml?txVariant=doku_mandiri_va", "merchantName": "YOUR_SHOP_NAME", "paymentMethodType": "doku_alfamart", "reference": "8520126030105485", "shopperEmail": "john.smith@adyen.com", "shopperName": "John Smith", "totalAmount": { "currency": "IDR", "value": 10000 }, "type": "voucher" } } -
After you show the voucher to the shopper, get the payment outcome.
Handle the redirect
If you get a redirect action.type
:
-
Get the
action.url
from the /payments response:/payments response for a redirect payment methodExpand viewCopy link to code blockCopy code{ "action": { "method": "GET", "paymentMethodType": "ideal", "type": "redirect", "url": "https://test.adyen.com/hpp/redirectIdeal.shtml?brandCode=ideal¤cyCode=EUR&issuerId=1121..." } } -
Redirect the shopper to the
action.url
with the HTTP method fromaction.method
.For redirect payment methods, the
action.method
is GET:Redirect payment method URLExpand viewCopy link to code blockCopy codecurl https://test.adyen.com/hpp/redirectIdeal.shtml?brandCode=ideal¤cyCode=EUR&issuerId=1121... \
The shopper is redirected to a page where they need to take additional action, depending on the payment method.
For security reasons, when displaying the redirect in the app, we recommend that you use SFSafariViewController for iOS or Chrome Custom Tabs for Android, instead of WebView objects. Also refer to the security best practices for WebView.
After the shopper completes the payment, they are redirected back to your
returnUrl
with HTTP GET. ThereturnUrl
is appended with a Base64-encodedredirectResult
:GET /?shopperOrder=12xy..&&redirectResult=X6XtfGC3%21Y... HTTP/1.1 Host: www.your-company.com/checkout -
Get the
redirectResult
appended to the URL and pass it to your server to verify the payment result in the next step.If a shopper completed the payment but failed to return to your website or app, you will receive the outcome of the payment in a webhook event.
-
From your server, make a /payments/details request, specifying:
details
: Object that contains the URL-decodedredirectResult
.
/payments/details requestExpand viewCopy link to code blockCopy codecurl https://checkout-test.adyen.com/checkout/v70/payments/details \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d '{ "details": { "redirectResult": "eyJ0cmFuc1N0YXR1cyI6IlkifQ==" } }' The response contains:
resultCode
: The current status of the payment.pspReference
: Our unique identifier for the transaction.
/payments/details responseExpand viewCopy link to code blockCopy code{ "resultCode": "Authorised", "pspReference": "NC6HT9CRT65ZGN82" }
Send additional payment details
If you redirected your shopper to another website, or if your shopper went through the native 3D Secure 2 flow, use the /payments/details endpoint to complete the payment.
From your server, make a POST /payments/details request specifying:
details
: Pass either URL-decodedredirectResult
from when the shopper returned to your website, or thethreeDSResult
from the 3D Secure 2 Component.
The following example shows how to submit additional payment details after a redirect:
curl https://checkout-test.adyen.com/checkout/v69/payments/details \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d '{ "details": { "redirectResult": "eyJ0cmFuc1N0YXR1cyI6IlkifQ==" } }'
The response includes:
pspReference
: Our unique identifier for the transaction.resultCode
: Indicates the current status of the payment.
{ "pspReference": "NC6HT9CRT65ZGN82", "resultCode": "Authorised" }
Get the payment outcome
After finishes the payment flow, you can show the shopper the current payment status. Adyen sends a webhook with the outcome of the payment.
Inform the shopper
Use the
resultCode
to show the shopper the current payment status. This synchronous response doesn't give you the final outcome of the payment. You get the final payment status in a webhook that you use to update your order management system.
Update your order management system
You get the outcome of each payment asynchronously, in an AUTHORISATION webhook. Use the merchantReference
from the webhook to match it to your order reference.
For a successful payment, the event contains success
: true.
{ "live": "false", "notificationItems":[ { "NotificationRequestItem":{ "eventCode":"AUTHORISATION", "merchantAccountCode":"YOUR_MERCHANT_ACCOUNT", "reason":"033899:1111:03/2030", "amount":{ "currency":"EUR", "value":2500 }, "operations":["CANCEL","CAPTURE","REFUND"], "success":"true", "paymentMethod":"mc", "additionalData":{ "expiryDate":"03/2030", "authCode":"033899", "cardBin":"411111", "cardSummary":"1111" }, "merchantReference":"YOUR_REFERENCE", "pspReference":"NC6HT9CRT65ZGN82", "eventDate":"2021-09-13T14:10:22+02:00" } } ] }
For an unsuccessful payment, you get success
: false, and the reason
field has details about why the payment was unsuccessful.
{ "live": "false", "notificationItems":[ { "NotificationRequestItem":{ "eventCode":"AUTHORISATION", "merchantAccountCode":"YOUR_MERCHANT_ACCOUNT", "reason":"validation 101 Invalid card number", "amount":{ "currency":"EUR", "value":2500 }, "success":"false", "paymentMethod":"unknowncard", "additionalData":{ "expiryDate":"03/2030", "cardBin":"411111", "cardSummary":"1112" }, "merchantReference":"YOUR_REFERENCE", "pspReference":"KHQC5N7G84BLNK43", "eventDate":"2021-09-13T14:14:05+02:00" } } ] }
Error handling
In case you encounter errors in your integration, refer to the following:
- API error codes: If you receive a non-HTTP 200 response, use the
errorCode
to troubleshoot and modify your request. - Payment refusals: If you receive an HTTP 200 response with an Error or Refused
resultCode
, check the refusal reason and, if possible, modify your request.
Test and go live
Before going live, use our list of test cards and other payment methods to test your integration. We recommend testing each payment method that you intend to offer to your shoppers.
You can check the status of a test payment in your Customer Area, under Transactions > Payments.
To debug or troubleshoot test payments, you can also use API logs in your test environment.
When you are ready to go live, you need to:
- Apply for a live account.
- Assess your PCI DSS compliance by submitting:
- the Self-Assessment Questionnaire-A, if you are using the Custom Card Component.
- the Self-Assessment Questionnaire-D, if you are submitting raw card data.
- Configure your live account.
- Submit a request to add payment methods in your live Customer Area .
- Switch from test to our live endpoints.
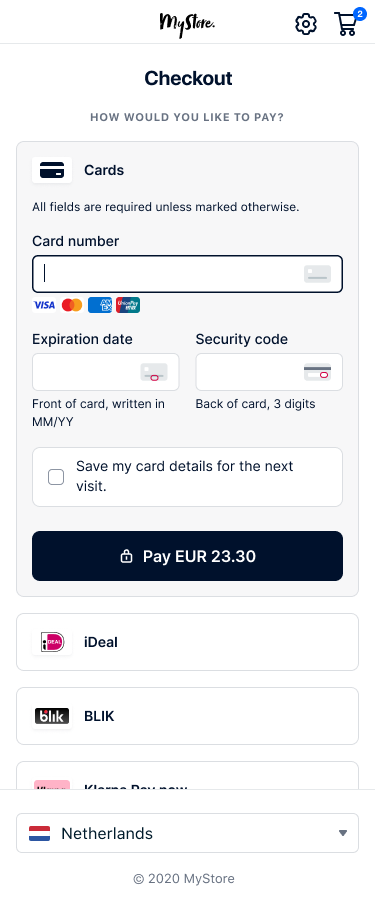
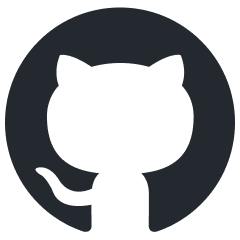
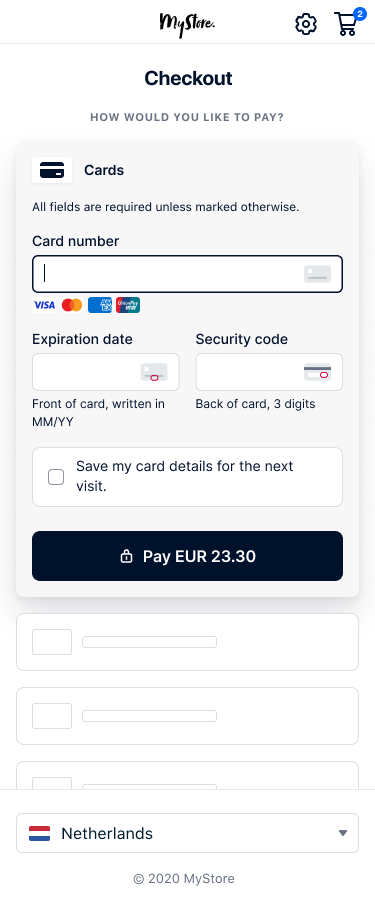
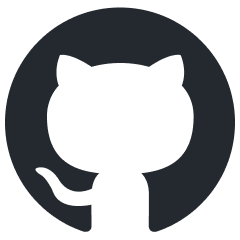
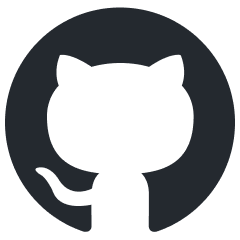
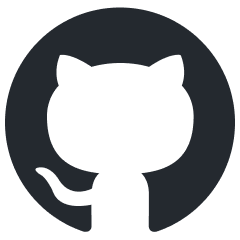
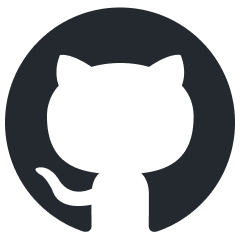
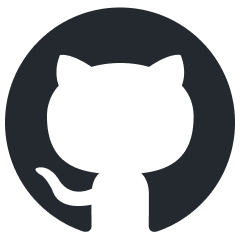
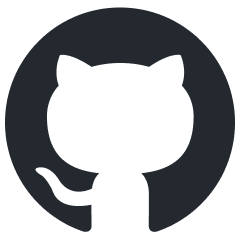
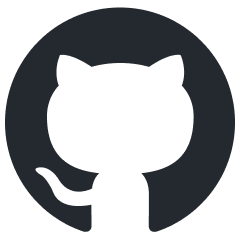
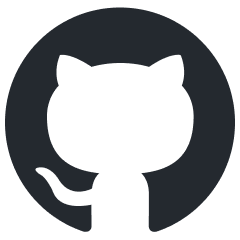
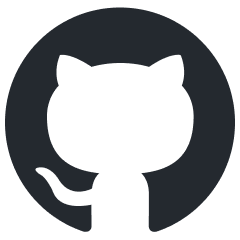