React Native Drop-in
Render a list of available payment methods anywhere in your app.
Supported payment methods
Cards, buy now pay later, wallets, and many more.
See all supported payment methods
Features
- Lowest development time to integrate payment methods
- UI styling customization for the list of payment methods
- Adding payment methods to the list requires no extra development time
- 3D Secure 2 support built in
Start integrating with React Native Drop-in
Choose your version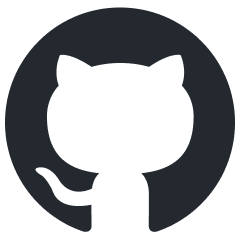
Adyen React Native on GitHub
View the Adyen React Native repository
View our example integrations
Drop-in is our pre-built UI solution for accepting payments in your app. Drop-in shows all payment methods as a list, in the same block. Your server makes API requests to the /paymentMethods, /payments, and /payments/details endpoints.
Requirements
Before you begin to integrate, make sure you have followed the Get started with Adyen guide to:
- Get an overview of the steps needed to accept live payments.
- Create your test account.
After you have created your test account:
- Get your API key.
- Get your client key.
- Set up webhooks to know the payment outcome.
How it works
For a Drop-in integration, you must implement the following parts:
If you are integrating these parts separately, you can start at the corresponding part of this integration guide:
The parts of your integration work together to complete the payment flow:
- The shopper goes to the checkout page.
- Your server uses the shopper's country and currency information from your client to get available payment methods.
- Drop-in shows the available payment methods, collects the shopper's payment details, handles additional actions, and shows the payment result to the shopper.
- Your webhook server receives the notification containing the payment outcome.
Install an API library
We provide server-side API libraries for several programming languages, available through common package managers, like Gradle and npm, for easier installation and version management. Our API libraries will save you development time, because they:
- Use an API version that is up to date.
- Have generated models to help you construct requests.
- Send the request to Adyen using their built-in HTTP client, so you do not have to create your own.
Try our example integration
Requirements
- Java 11 or later.
Installation
You can use Maven, adding this dependency to your project's POM.
<dependency> <groupId>com.adyen</groupId> <artifactId>adyen-java-api-library</artifactId> <version>LATEST_VERSION</version> </dependency>
You can find the latest version on GitHub. Alternatively, you can download the release on GitHub.
Setting up the client
Create a singleton resource that you use for the API requests to Adyen:
// Import the required classes. package com.adyen.service; import com.adyen.Client; import com.adyen.service.checkout.PaymentsApi; import com.adyen.model.checkout.Amount; import com.adyen.enums.Environment; import com.adyen.service.exception.ApiException; import java.io.IOException; public class Snippet { public Snippet() throws IOException, ApiException { // Set up the client and service. Client client = new Client("ADYEN_API_KEY", Environment.TEST); } }
Get available payment methods
When your shopper is ready to pay, get a list of the available payment methods based on their country, device, and the payment amount.
From your server, make a POST /paymentMethods request, providing the following parameters. While most parameters are optional, we recommend that you include them because Adyen uses these to tailor the list of payment methods for your shopper.
We use the optional parameters to tailor the list of available payment methods to your shopper.
Parameter name | Required | Description |
---|---|---|
merchantAccount |
![]() |
Your merchant account name. |
amount |
The currency of the payment and its value in minor units. |
|
channel |
The platform where the payment is taking place. For example, when you set this to iOS, Adyen returns only the payment methods available for iOS. | |
countryCode |
The shopper's country/region. Adyen returns only the payment methods available in this country. Format: the two-letter ISO-3166-1 alpha-2 country code. Exception: QZ (Kosovo). |
|
shopperLocale |
By default, the shopperlocale is set to en-US. To change the language, set this to the shopper's language and country code. The front end also uses this locale. |
For example, to get the available payment methods for a shopper in the Netherlands, for a payment of EUR 10:
curl https://checkout-test.adyen.com/checkout/v70/paymentMethods \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d '{ "merchantAccount": "ADYEN_MERCHANT_ACCOUNT", "countryCode": "NL", "amount": { "currency": "EUR", "value": 1000 }, "channel": "Android", "shopperLocale": "nl-NL" }'
The response includes the list of available paymentMethods
:
{ "paymentMethods":[ { "details":[...], "name":"Cards", "type":"scheme" ... }, { "details":[...], "name":"SEPA Direct Debit", "type":"sepadirectdebit" }, ... ] }
Pass the response to your client app. Use this in the next step to show available payment methods to the shopper.
Add Adyen Drop-in to your app
1. Add Adyen React Native to your project
$ yarn add @adyen/react-native
2. Install
- Run
pod install
. -
In your
AppDelegate.m
file, add a return URL handler for handling redirects from other apps. For example:iOS return URL handlerExpand viewCopy link to code blockCopy codeimport <adyen-react-native/ADYRedirectComponent.h> { ... - (BOOL)application:(UIApplication *)app openURL:(NSURL *)url options:(NSDictionary<UIApplicationOpenURLOptionsKey,id> *)options { return [ADYRedirectComponent applicationDidOpenURL:url]; } If you use
RCTLinkingManager
or other ways of deep linking, useADYRedirectComponent.applicationDidOpenURL
first:Return URL handler with deep linkingExpand viewCopy link to code blockCopy codeimport <adyen-react-native/ADYRedirectComponent.h> { ... - (BOOL)application:(UIApplication *)app openURL:(NSURL *)url options:(NSDictionary<UIApplicationOpenURLOptionsKey,id> *)options { return [ADYRedirectComponent applicationDidOpenURL:url] || [super application:application openURL:url options:options] || [RCTLinkingManager application:application openURL:url options:options]; } If you want to support universal links in your app, add the following return URL handler instead:
Return handler with universal link supportExpand viewCopy link to code blockCopy codeimport <adyen-react-native/ADYRedirectComponent.h> { ... - (BOOL)application:(UIApplication *)application continueUserActivity:(nonnull NSUserActivity *)userActivity restorationHandler:(nonnull void (^)(NSArray<id<UIUserActivityRestoring>> * _Nullable))restorationHandler { if ([[userActivity activityType] isEqualToString:NSUserActivityTypeBrowsingWeb]) { NSURL *url = [userActivity webpageURL]; if (![url isEqual:[NSNull null]] && [ADYRedirectComponent applicationDidOpenURL:url]) { return YES; } } BOOL result = [RCTLinkingManager application:application continueUserActivity:userActivity restorationHandler:restorationHandler]; return [super application:application continueUserActivity:userActivity restorationHandler:restorationHandler] || result; }
If your Podfile
has use_frameworks!
, import the redirect component using underscores (_) instead of hyphens(-):
#import <adyen_react_native/ADYRedirectComponent.h>
3. Create a configuration object
Create a configuration object with the following properties:
Parameter | Required | Description | |
---|---|---|---|
environment |
![]() |
Use test. When you're ready to accept live payments, change the value to one of our live environments. | |
clientKey |
![]() |
A public key linked to your API credential, used for client-side authentication. | |
returnUrl |
![]() |
For iOS, this is the URL to your app, where the shopper should return, after a redirection. Maximum of 1024 characters. For more information on setting a custom URL scheme for your app, read the Apple Developer documentation. For Android, this value is automatically overridden by AdyenCheckout . |
|
countryCode |
If you want to show the amount on the Pay button. | The shopper's country/region. Format: the two-letter ISO-3166-1 alpha-2 country code. Exception: QZ (Kosovo). |
|
amount |
If you want to show the amount on the Pay button. | The currency and value of the payment, in minor units. |
For example:
const configuration = { // When you're ready to accept live payments, change the value to one of our live environments. environment: 'test', clientKey: 'YOUR_CLIENT_KEY', // For iOS, this is the URL to your app. For Android, this is automatically overridden by AdyenCheckout. returnUrl: 'your-app://', // Must be included to show the amount on the Pay button. countryCode: 'NL', amount: { currency: 'EUR', value: 1000 } };
To add configuration for specific payment methods, add these in a payment method specific configuration object. For example, for Apple Pay:
const configuration: Configuration = { environment: 'test', // When you're ready to accept real payments, change the value to a suitable live environment. clientKey: 'YOUR_CLIENT_KEY', returnUrl: 'your-app://', countryCode: 'NL', amount: { currency: 'EUR', value: 1000 }, applepay: { merchantID: 'APPLE_PAY_MERCHANT_ID', merchantName: 'APPLE_PAY_MERCHANT_NAME' } };
Optional configuration
Optionally, you can configure the following properties for Drop-in.
Parameter | Description |
---|---|
showPreselectedStoredPaymentMethod |
When enabled, shows the preselected stored payment method view. Defaults to true. |
skipListWhenSinglePaymentMethod |
Set to true to skip showing the payment methods list when only one non-instant payment method is available. Defaults to false. |
title Only for iOS |
Set a custom title for the pre-selected stored payment view. By default, the app's name is used. |
showRemovePaymentMethodButton |
Allows the shopper to remove a stored payment method. Defaults to false. When enabled, you must also implement the onDisableStoredPaymentMethod callback. |
onDisableStoredPaymentMethod(storedPaymentMethod, resolve, reject) => {} |
Called when a shopper removes a stored payment method. To remove the selected payment method, make a /disable request using the storedPaymentMethodId . Then call either resolve() or reject() , depending on the /disable response. |
For example:
const configuration: Configuration = { environment: 'test', // When you're ready to accept real payments, change the value to a suitable live environment. clientKey: '{YOUR_CLIENT_KEY}', returnUrl: 'your-app://', countryCode: 'NL', amount: { currency: 'EUR', value: 1000 }, dropin: { skipListWhenSinglePaymentMethod: true, showPreselectedStoredPaymentMethod: false } };
4. Initialize Drop-in
- Configure
AdyenCheckout
, setting the following:
Parameter | Description |
---|---|
config |
Your configuration object. |
paymentMethods |
The full response from the /paymentMethods endpoint. |
onSubmit |
Callback that uses data to make a /payments request when the shopper selects the Pay button and the payment details are valid. |
onAdditionalDetails |
Callback that uses data to make a /payments/details request when a payment requires additional details, for example to authenticate with 3D Secure or to pay using a QR code. |
onError |
Callback that handles errors. |
In your onSubmit
, onAdditionalDetails
, and onError
callbacks, you must call component.hide(result)
to dismiss the payment UI when the API request is completed and the payment result is known.
For example:
// Import AdyenCheckout. import { AdyenCheckout } from '@adyen/react-native'; import { useCallback } from 'react'; const submitHandler = useCallback( (data, component, extra) => { // Make a /payments request. // When this callback is executed, you must call `component.hide(true | false)` to dismiss the payment UI. }, [...], ); const errorHandler = useCallback( (error, component) => { // Handle errors or termination by shopper. // When this callback is executed, you must call `component.hide(false)` to dismiss the payment UI. }, [...], ); const additionalDetailsHandler = useCallback( (data, component) => { // Make a /payments/details request. // When this callback is executed, you must call `component.hide(true | false)` to dismiss the payment UI. }, [...], ); <AdyenCheckout config={configuration} paymentMethods={paymentMethods} onSubmit={submitHandler} onError={errorHandler} onAdditionalDetails={additionalDetailsHandler} > <YourCheckoutView/> </AdyenCheckout>
- Create a way, like a button, for
AdyenCheckout
to call thestart
function.
// Import useAdyenCheckout. import { useAdyenCheckout } from '@adyen/react-native'; // Set your View to use AdyenCheckout as the context. const YourCheckoutView = () => { const { start } = useAdyenCheckout(); return ( // Create a way, like a checkout button, that starts Drop-in. <Button title="Checkout" // Use dropIn to show the full list of available payment methods. onPress={() => { start('dropIn'); }} /> ); };
The context starts Adyen's Native Component for Drop-in.
Make a payment
When the shopper selects the Pay button or chooses to pay with a payment method that requires a redirection, the Native Component calls onSubmit
, and you make a payment request to Adyen.
From your server, make a POST /payments request specifying:
Parameter name | Required | Description |
---|---|---|
merchantAccount |
![]() |
Your merchant account name. |
amount |
![]() |
The currency of the payment and its value in minor units. |
reference |
![]() |
Your unique reference for this payment. |
paymentMethod |
![]() |
The payment method from the payload of the onSubmit event from your client app. |
returnUrl |
![]() For Android, this value is automatically overridden by AdyenCheckout . |
For iOS, this is the URL to your app, where the shopper should return, after a redirection. Maximum of 1024 characters. For more information on setting a custom URL scheme for your app, read the Apple Developer documentation. If the URL to return to includes non-ASCII characters, like spaces or special letters, URL encode the value. The URL must not include personally identifiable information (PII), for example name or email address. |
applicationInfo
|
If you are building an Adyen solution for multiple merchants, include some basic identifying information, so that we can offer you better support. For more information, refer to Building Adyen solutions. |
Include additional parameters in your payment request to:
- Integrate some payment methods. For more information, refer to our payment method integration guides.
- Make use of our risk management features. For more information, see Required risk fields.
- Use native 3D Secure 2 authentication.
- Tokenize your shopper's payment details or make recurring payments.
The following example shows how to make a payment request for EUR 10:
curl https://checkout-test.adyen.com/checkout/v70/payments \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d '{ "amount":{ "currency":"EUR", "value":1000 }, "reference":"YOUR_ORDER_NUMBER", "paymentMethod":STATE_DATApaymentMethod field of an object passed from your client app, "returnUrl":"my-app://adyen", "merchantAccount":"ADYEN_MERCHANT_ACCOUNT" }'
Your next steps depend on if the /payments response contains an action
object:
Description | Next steps | |
---|---|---|
No action object |
No additional steps are needed to complete the payment. | Get the payment outcome. |
With action object |
The shopper needs to do additional actions to complete the payment. | 1. Pass the action object to your client app. The Native Component uses this to handle the required action. 2. Handle the additional action. |
The following example shows the /payments response for iDEAL, a redirect payment method.
{ "resultCode": "RedirectShopper", "action": { "paymentMethodType": "ideal", "url": "https://checkoutshopper-test.adyen.com/checkoutshopper/checkoutPaymentRedirect?redirectData=X6Xtf...", "method": "GET", "type": "redirect" } }
Errors
If an error occurs, you get an error object from the onError
callback with one of the following error codes:
Error code | Description | Action to take |
---|---|---|
canceledByShopper | The shopper canceled the payment. | Take the shopper back to the checkout page. |
notSupported | The payment method isn't supported by the shopper's device. | Tell the shopper that the payment method isn't supported by their device. |
noClientKey | No clientKey configured. |
Tell the shopper that an error occurred. |
noPayment | No payment information configured. |
Tell the shopper that an error occurred. |
invalidPaymentMethods | Can't parse the paymentMethods list, or the list is empty. |
Tell the shopper that an error occurred. |
noPaymentMethod | Can't find the selected payment method. | Tell the shopper that their selected payment method is currently unavailable. |
Handle the additional action
Some payment methods require additional action from the shopper such as: to scan a QR code, to authenticate a payment with 3D Secure, or to log in to their bank's website to complete the payment.
To handle these additional front-end actions, the AdyenCheckout
provides a handle
function to perform the next step depending on the action.type
.
action.type |
Description | Next steps |
---|---|---|
voucher | Drop-in shows the voucher which the shopper uses to complete the payment. |
Get the payment outcome. |
redirect | Drop-in redirects the shopper to another website or app to complete the payment. | 1. When the shopper returns to your website, your server needs to handle the redirect result. 2. Send additional payment details to check the payment result. |
qrCode | Drop-in shows the QR code and calls the onAdditionalDetails event. |
1. Get the data from the onAdditionalDetails event and pass it your server. 2. Send additional payment details to check the payment result. |
await | Drop-in shows the waiting screen while the shopper completes the payment. Depending on the payment outcome, Drop-in calls the onAdditionalDetails or onError event. |
1. Get the data from the onAdditionalDetails or onError event and pass it your server. 2. Send additional payment details to check the payment result. |
sdk | Drop-in shows the specific payment method's UI as an overlay and calls the onAdditionalDetails event. |
1. Get the data from the onAdditionalDetails event and pass it your server. 2. Send additional payment details to check the payment result. |
threeDS2 | The payment qualifies for 3D Secure 2 and goes through the authentication flow. Drop-in performs the authentication flow, and calls the onAdditionalDetails event. |
1. Get the data from the onAdditionalDetails event and pass it your server. 2. Send additional payment details to complete the payment. |
Call handle
from one of Adyen's Native Components and pass the action
to. For example:
// Call the handle function from the Native Component corresponding to the payment method. nativeComponent.handle(action)
Handle the redirect result
If you receive an action.type
redirect, Drop-in redirects your shopper to another website to complete the payment.
After completing the payment, the shopper is redirected back to your returnUrl
with an HTTP GET. The returnUrl
is appended with a Base64-encoded redirectResult
:
GET /?shopperOrder=12xy..&&redirectResult=X6XtfGC3%21Y... HTTP/1.1 Host: your-app://checkout
- URL-decode the
redirectResult
and pass it to your server. - Send additional payment details.
If a shopper completed the payment but failed to return to your website, wait for the webhook to know the payment result.
Send additional payment details
If the shopper performed additional action to complete the payment, you need to make another request to Adyen to either submit the additional payment details or to check the payment result.
From your server, make a POST /payments/details request with the data
from the didProvide
method from your client app.
curl https://checkout-test.adyen.com/checkout/v70/payments/details \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d 'STATE_DATAobject passed from your client app'
Your next steps depend on whether the /payments/details response contains an action
object:
Description | Next steps | |
---|---|---|
No action object |
No additional steps are needed to complete the payment. This is always the case for Checkout API v67 and above. |
Get the payment outcome. |
action object |
The shopper needs to do additional actions to complete the payment. | 1. Pass the action object to your client app. 2. Handle the additional action again. |
The response includes:
pspReference
: Adyen's unique identifier for the transaction.resultCode
: Indicates the current status of the payment.
{ "pspReference": "NC6HT9CRT65ZGN82", "resultCode": "Authorised" }
{ "pspReference": "KHQC5N7G84BLNK43", "refusalReason": "Not enough balance", "resultCode": "Refused" }
Get the payment outcome
After Drop-in finishes the payment flow, you can show the shopper the current payment status. Adyen sends a webhook with the outcome of the payment.
Inform the shopper
Use the
resultCode
to show the shopper the current payment status. This synchronous response doesn't give you the final outcome of the payment. You get the final payment status in a webhook that you use to update your order management system.
Update your order management system
You get the outcome of each payment asynchronously, in an AUTHORISATION webhook. Use the merchantReference
from the webhook to match it to your order reference.
For a successful payment, the event contains success
: true.
{ "live": "false", "notificationItems":[ { "NotificationRequestItem":{ "eventCode":"AUTHORISATION", "merchantAccountCode":"YOUR_MERCHANT_ACCOUNT", "reason":"033899:1111:03/2030", "amount":{ "currency":"EUR", "value":2500 }, "operations":["CANCEL","CAPTURE","REFUND"], "success":"true", "paymentMethod":"mc", "additionalData":{ "expiryDate":"03/2030", "authCode":"033899", "cardBin":"411111", "cardSummary":"1111" }, "merchantReference":"YOUR_REFERENCE", "pspReference":"NC6HT9CRT65ZGN82", "eventDate":"2021-09-13T14:10:22+02:00" } } ] }
For an unsuccessful payment, you get success
: false, and the reason
field has details about why the payment was unsuccessful.
{ "live": "false", "notificationItems":[ { "NotificationRequestItem":{ "eventCode":"AUTHORISATION", "merchantAccountCode":"YOUR_MERCHANT_ACCOUNT", "reason":"validation 101 Invalid card number", "amount":{ "currency":"EUR", "value":2500 }, "success":"false", "paymentMethod":"unknowncard", "additionalData":{ "expiryDate":"03/2030", "cardBin":"411111", "cardSummary":"1112" }, "merchantReference":"YOUR_REFERENCE", "pspReference":"KHQC5N7G84BLNK43", "eventDate":"2021-09-13T14:14:05+02:00" } } ] }
Test and go live
Before going live, use our list of test cards and other payment methods to test your integration. We recommend testing each payment method that you intend to offer to your shoppers.
You can check the status of a test payment in your Customer Area, under Transactions > Payments.
To debug or troubleshoot test payments, you can also use API logs in your test environment.
When you are ready to go live, you need to:
- Apply for a live account. Review the process to start accepting payments on Get started with Adyen.
- Assess your PCI DSS compliance by submitting the Self-Assessment Questionnaire-A.
- Configure your live account.
- Submit a request to add payment methods in your live Customer Area .
- Switch from test to our live endpoints.
- Load Drop-in from one of our live environments and set the
environment
to match your live endpoints:
Endpoint region | environment value |
---|---|
Europe | live |
Australia | live-au |
US | live-us |
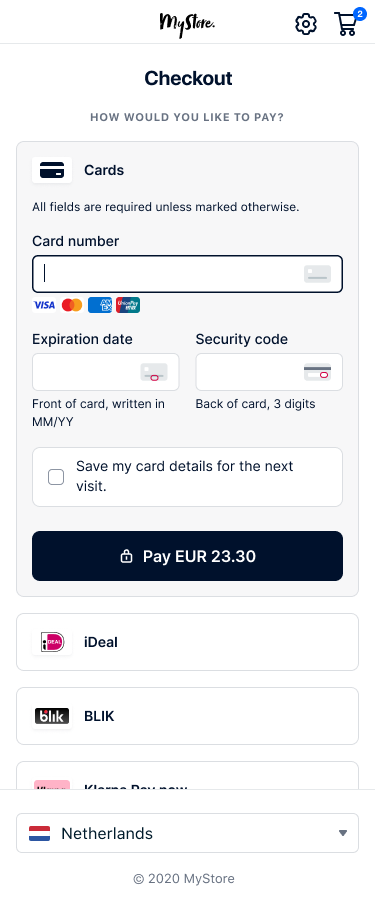
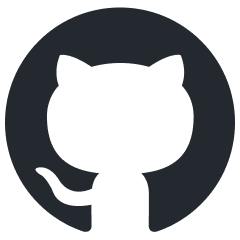
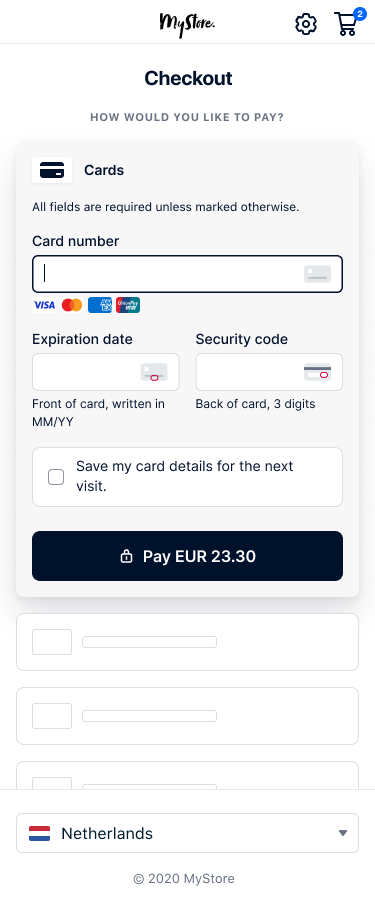
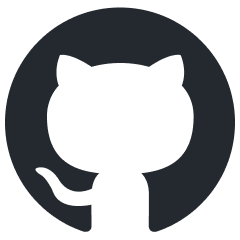
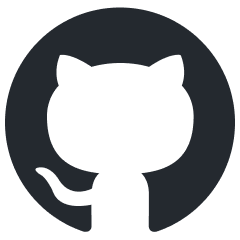
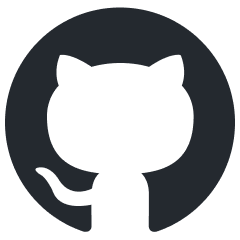
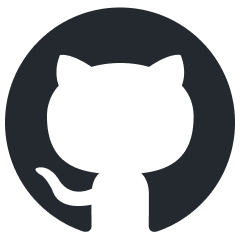
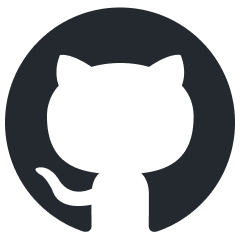
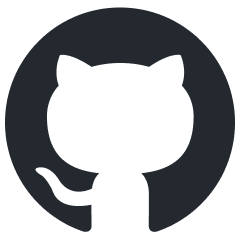
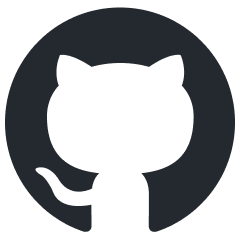
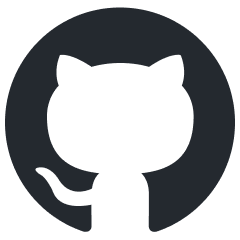
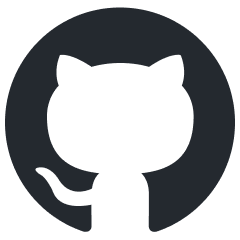