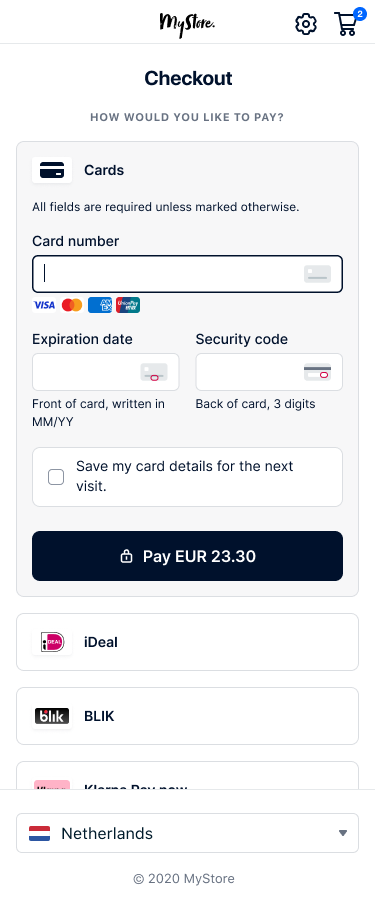
Web Drop-in
Render a list of available payment methods anywhere on your website.
Supported payment methods
Cards, buy now pay later, wallets, and many more.
See all supported payment methods
Features
- Low development time to integrate payment methods
- UI styling customization for the list of payment methods
- Adding payment methods to the list requires no extra development time
- 3D Secure 2 support built in
Demo
Start integrating with Web Drop-in
Choose your versionDrop-in is our pre-built UI solution for accepting payments on your website. Drop-in shows all payment methods as a list, in the same block. This integration requires you to make API requests to /paymentMethods, /payments, and /payments/details endpoints.
Adding new payment methods usually doesn't require more development work. Drop-in supports cards, wallets, and most local payment methods.
Introducing Web v6
Improvements
The Web v6 library introduces the following improvements:
- Reduced bundle size through tree shaking
- Enhanced design
- Enhanced Typescript developer experience
- Better alignment of express payment methods
- Added support for 6 localizations
- Support for Apple Pay Order tracking
- Improve AVS checks for Google Pay and Apple Pay
To update your existing integration, see Migrate to Adyen Web v6.
Requirements
Check out our Node.js + Express tutorial
Follow our tutorial to integrate Drop-in with Node.js and Express.
Before you begin to integrate, make sure you have followed the Get started with Adyen guide to:
- Get an overview of the steps needed to accept live payments.
- Create your test account.
After you have created your test account:
- Get your API key.
- Get your client key.
- Set up webhooks to know the payment outcome.
To make sure that your 3D Secure integration works on Chrome, your cookies need to have the SameSite attribute. For more information, refer to Chrome SameSite Cookie policy.
How it works
For a Drop-in integration, you must implement the following parts:
If you are integrating these parts separately, you can start at the corresponding part of this integration guide:
The parts of your integration work together to complete the payment flow:
- From your server, submit a request to get a list of payment methods available to the shopper.
- Create an instance of Drop-in on your payments form.
- From your server, submit a payment request with data you receive from Drop-in.
- Determine from the response if you need to perform additional actions on your client website.
- From your server, submit additional payment details with data you receive from Drop-in.
- Present the payment result to the shopper.
When you have completed the integration, proceed to test your integration.
Install an API library
We provide server-side API libraries for several programming languages, available through common package managers, like Gradle and npm, for easier installation and version management. Our API libraries will save you development time, because they:
- Use an API version that is up to date.
- Have generated models to help you construct requests.
- Send the request to Adyen using their built-in HTTP client, so you do not have to create your own.
Try our example integration
Requirements
- Java 11 or later.
Installation
You can use Maven, adding this dependency to your project's POM.
<dependency> <groupId>com.adyen</groupId> <artifactId>adyen-java-api-library</artifactId> <version>LATEST_VERSION</version> </dependency>
You can find the latest version on GitHub. Alternatively, you can download the release on GitHub.
Setting up the client
Create a singleton resource that you use for the API requests to Adyen:
// Import the required classes. package com.adyen.service; import com.adyen.Client; import com.adyen.service.checkout.PaymentsApi; import com.adyen.model.checkout.Amount; import com.adyen.enums.Environment; import com.adyen.service.exception.ApiException; import java.io.IOException; public class Snippet { public Snippet() throws IOException, ApiException { // Set up the client and service. Client client = new Client("ADYEN_API_KEY", Environment.TEST); } }
Get available payment methods
When your shopper is ready to pay, get a list of the available payment methods based on their country, device, and the payment amount.
From your server, make a /paymentMethods request, specifying:
Parameter name | Required | Description |
---|---|---|
merchantAccount |
![]() |
Your merchant account name. |
amount |
The currency and value of the payment, in minor units. This is used to filter the list of available payment methods to your shopper. |
|
channel |
The platform of the shopper's device; use Web. This is used to filter the list of available payment methods to your shopper. | |
countryCode |
The shopper's country/region. This is used to filter the list of available payment methods to your shopper. Format: the two-letter ISO-3166-1 alpha-2 country code. Exception: QZ (Kosovo). |
|
shopperLocale |
By default, the shopperlocale is set to en-US. To change the language, set this to the shopper's language and country code. You also need to set the same locale within your Drop-in configuration. |
You can also include other optional parameters, for example allowedPaymentMethods or blockedPaymentMethods to customize which payment methods will be shown to the shopper.
The following example shows how to get the available payment methods for a shopper in the Netherlands, for a payment of EUR 10:
curl https://checkout-test.adyen.com/checkout/v71/paymentMethods \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d '{ "merchantAccount": "ADYEN_MERCHANT_ACCOUNT", "countryCode": "NL", "amount": { "currency": "EUR", "value": 1000 }, "channel": "Web", "shopperLocale": "nl-NL" }'
The response includes the list of available paymentMethods
:
{ "paymentMethods":[ { "details":[...], "name":"Cards", "type":"scheme" ... }, { "details":[...], "name":"SEPA Direct Debit", "type":"sepadirectdebit" }, ... ] }
Pass the response to your client website. You'll use this in the next step to show which payment methods are available for the shopper.
Add Drop-in to your payments form
Next, use Drop-in to show the available payment methods, and to collect payment details from your shopper.
Import using the Adyen Web npm package, or embed the Adyen Web script and stylesheet into your HTML file:
We offer two ways of importing with npm:
- Import Drop-in with all payment methods: this method resembles what you are used to before v6. You do not need to import individual payment methods, at the cost of a larger bundle size.
- Import Drop-in with individual payment methods: this method uses tree shaking to let you import only the payment methods you use, speeding up loading time, at the cost of maintainability (adding payment methods requires developer resources).
Import Drop-in with all payment methods
Install the Adyen Web Node package:
npm install @adyen/adyen-web --save
Import Adyen Web into your application:
import { AdyenCheckout, Dropin } from '@adyen/adyen-web/auto'; import '@adyen/adyen-web/styles/adyen.css';
Import Drop-in with individual payment methods
Install the Adyen Web Node package:
npm install @adyen/adyen-web --save
Import Adyen Web into your application.
import { AdyenCheckout, Dropin, Card, GooglePay, PayPal } from '@adyen/adyen-web'; import '@adyen/adyen-web/styles/adyen.css';
You can add your own styling by overriding the rules in this CSS file.
After you have imported the Adyen Web library, do the following:
-
Create a Document Object Model (DOM) element on your checkout page and put it where you want Drop-in to render on the page.
Expand viewCopy link to code blockCopy code<div id="dropin-container"></div>
If you are using JavaScript frameworks such as Vue or React, make sure that you use references instead of selectors and that you do not re-render the DOM element.
-
Create a global configuration object with the following parameters and events:
Parameter name Required Description paymentMethodsResponse
The full /paymentMethods
response returned when you get available payment methods.clientKey
A public key linked to your API credential, used for client-side authentication.
Web Drop-in versions before 3.10.1 useoriginKey
instead. Find out how to migrate from usingoriginKey
toclientKey
.locale
The shopper's locale. This is used to set the language rendered in the UI. For a list of supported locales, see Language and localization. countryCode
The shopper's country/region. This is used to filter the list of available payment methods to your shopper.
Format: the two-letter ISO-3166-1 alpha-2 country code. Exception: QZ (Kosovo).environment
Use test. When you are ready to accept live payments, change the value to one of our live environments. secondaryAmount
Shows the payment amount in an additional currency on the Pay button. You must do the currency conversion and set the amount.
This object has properties:currency
: The three-character ISO currency code.value
: The amount of the transaction, in minor units.currencyDisplay
: Sets the currency formatting. Default: symbol.
showPayButton
Shows or hides a Pay Button for each payment method. Defaults to true.
When set to false, you must override it inpaymentMethodsConfiguration
.
The Pay button triggers theonSubmit
event when payment details are valid. If you want to disable the button and then trigger the submit flow on your own, set this to false and call the.submit()
method from your own button implementation.
PayPal Smart Payment Buttons doesn't support the.submit()
method.amount
Amount to be displayed on the Pay Button. It expects an object with the value and currency properties. For example, { value: 1000, currency: 'USD' }
.Event name Required Description onSubmit(state, dropin, actions)
Create an event handler for this event, which is called when the shopper selects the Pay button, and the details are valid. This applies if the showPayButton
configuration parameter is set to true.
Makes a POST /payments request.
If the /payments request from your server is successful, you must callactions.resolve()
, passingresultCode
,action
, andorder
objects (if available) from the API response. You must call it even if the payment is unsuccessful.
If the /payments request from your server fails, or if an unexpected error occurs, callactions.reject()
.onAdditionalDetails(state, dropin, actions)
Create an event handler, called when a payment method requires more details, for example for native 3D Secure 2, or native QR code payment methods. Makes a POST /payments/details request.
If the /payments/details request from your server is successful, you must callactions.resolve()
, passingresultCode
,action
, andorder
objects (if available) from the API response. You must call it even if the payment is unsuccessful.
If the /payments/details request from your server fails, or if an unexpected error occurs, callactions.reject
.onPaymentCompleted(result, component)
Create an event handler, called when the payment is completed. onPaymentFailed(result, component)
Create an event handler, called when the payment failed. A failed payment has result code Cancelled, Error or Refused. onError(error)
Create an event handler, called when an error occurs in Drop-in. onChange(state, dropin)
Create an event handler, called when the shopper provides the required payment details. onActionHandled
Create an event handler, called when an action, for example a QR code or 3D Secure 2 authentication screen, is shown to the shopper. The following action.type
values trigger this callback:threeDS
qr
await
componentType
: The type of component that shows the action to the shopper.actionDescription
: A description of the action shown to the shopper.
Create a configuration objectExpand viewCopy link to code blockCopy codeconst configuration = { clientKey: "YOUR_CLIENT_KEY", environment: "test", amount: { value: 1000, currency: 'EUR' }, locale: 'nl-NL', countryCode: 'NL', // The full /paymentMethods response object from your server. Contains the payment methods configured in your account. paymentMethodsResponse: paymentMethodsResponse, onSubmit: async (state, component, actions) => { try { // Make a POST /payments request from your server. const result = await makePaymentsCall(state.data, countryCode, locale, amount); // If the /payments request from your server fails, or if an unexpected error occurs. if (!result.resultCode) { actions.reject(); return; } const { resultCode, action, order, donationToken } = result; // If the /payments request request form your server is successful, you must call this to resolve whichever of the listed objects are available. // You must call this, even if the result of the payment is unsuccessful. actions.resolve({ resultCode, action, order, donationToken, }); } catch (error) { console.error("onSubmit", error); actions.reject(); } }, onAdditionalDetails: async (state, component, actions) => { try { // Make a POST /payments/details request from your server. const result = await makeDetailsCall(state.data); // If the /payments/details request from your server fails, or if an unexpected error occurs. if (!result.resultCode) { actions.reject(); return; } const { resultCode, action, order, donationToken } = result; // If the /payments/details request request from your server is successful, you must call this to resolve whichever of the listed objects are available. // You must call this, even if the result of the payment is unsuccessful. actions.resolve({ resultCode, action, order, donationToken, }); } catch (error) { console.error("onSubmit", error); actions.reject(); } }, onPaymentCompleted: (result, component) => { console.info(result, component); }, onPaymentFailed: (result, component) => { console.info(result, component); }, onError: (error, component) => { console.error(error.name, error.message, error.stack, component); } ); -
Create an instance of
AdyenCheckout
, passing the global configuration object you created:Expand viewCopy link to code blockCopy codeconst checkout = await AdyenCheckout(configuration);
-
Create and mount an instance of
Dropin
, passing the instance ofAdyenCheckout
you created. You can add optional Drop-in configuration your instance ofDropin
. How you create it depends on if you import resources for all payment methods or individual payment methods.checkout.jsExpand viewCopy link to code blockCopy code// Create an instance of Drop-in and mount it to the container you created. const dropin = new Dropin(checkout).mount('#dropin'); When the shopper selects the Pay button, Drop-in calls the
onSubmit
event, which contains astate.data
. These are the shopper details that you need to make the payment. -
Pass the
state.data
to your server.Sample state from onSubmit event for a card paymentExpand viewCopy link to code blockCopy code{ isValid: true, data: { paymentMethod: { type: "scheme", encryptedCardNumber: "adyenjs_0_1_18$k7s65M5V0KdPxTErhBIPoMPI8HlC..", encryptedExpiryMonth: "adyenjs_0_1_18$p2OZxW2XmwAA8C1Avxm3G9UB6e4..", encryptedExpiryYear: "adyenjs_0_1_18$CkCOLYZsdqpxGjrALWHj3QoGHqe+..", encryptedSecurityCode: "adyenjs_0_1_18$XUyMJyHebrra/TpSda9fha978+.." holderName: "S. Hopper" } } }
Optional Drop-in configuration
You can add the following configuration parameters to the Dropin
configuration:
Parameter name | Description |
---|---|
openFirstPaymentMethod |
When enabled, Drop-in opens the first payment method automatically on page load. Defaults to true. |
openFirstStoredPaymentMethod |
When enabled, Drop-in opens the payment method with stored card details on page load. This option takes precedence over openFirstPaymentMethod . Defaults to true. |
openPaymentMethod.type |
Automatically selects the specified payment method when Drop-in renders. Set the payment method type that you want to be automatically selected as the value. |
showStoredPaymentMethods |
Shows or hides payment methods with stored card details. Defaults to true. |
showRemovePaymentMethodButton |
Allows the shopper to remove a stored payment method. Defaults to false. If using this prop, you must also implement the onDisableStoredPaymentMethod callback. |
showPaymentMethods |
Shows or hides regular (not stored) payment methods. Set to false if you only want to show payment methods with stored card details. Defaults to true. |
paymentMethodsConfiguration |
Configuration for individual payment methods. The payment method guides have configuration options specific to each payment method. If you include this in the configuration on your instance of DropIn , it overrides global payment method configuration on your instance of AdyenCheckout . |
redirectFromTopWhenInIframe |
If your Drop-in is inside of an iframe element, set to true if you want redirects to be performed on the top-level window. We recommend that you do not put Component in an iframe. |
instantPaymentTypes |
Moves payment methods to the top of the list of available payment methods. This is available for Apple Pay and Google Pay. |
disableFinalAnimation |
When enabled, disables the final animation after a shopper completes the payment (whether successful or failed). This lets you implement your own Defaults to false. |
showRadioButton |
When enabled, payment methods in the Drop-in have a radio button. Defaults to false. |
Events
Use the following events to include additional logic on your checkout page:
Event name | Description |
---|---|
onReady() |
Called when Drop-in is initialized and ready for use. |
onSelect(component) |
Called when the shopper selects a payment method. |
onDisableStoredPaymentMethod(storedPaymentMethodId, resolve, reject) |
Called when a shopper removes a stored payment method. To remove the selected payment method, make a DELETE /storedPaymentMethod request including the storedPaymentMethodId . Then call either resolve() or reject() , depending on the /storedPaymentMethod response. |
Methods
Drop-in supports the following methods:
Method name | Description |
---|---|
mount(selector) |
Mounts the Drop-in into the DOM returned by the selector . The selector must be either a valid CSS selector string or an HTMLElement reference. |
unmount() |
Unmounts the Drop-in from the DOM. We recommend to unmount in case the payment amount changes after the initial mount. |
closeActivePaymentMethod() |
Closes a selected payment method, for example if you want to reset the Drop-in. |
Make a payment
After the shopper selects the Pay button or chooses to pay with a payment method that requires a redirection, you need to make a payment request to Adyen.
From your server, make a /payments request specifying:
Parameter name | Required | Description |
---|---|---|
merchantAccount |
![]() |
Your merchant account name. |
amount |
![]() |
The currency of the payment and its value in minor units. |
reference |
![]() |
Your unique reference for this payment. |
paymentMethod |
![]() |
The state.data.paymentMethod from the onSubmit event from your client website. |
returnUrl |
![]() |
URL to where the shopper should be taken back to after a redirection. The URL can contain a maximum of 1024 characters and should include the protocol: http:// or https:// . You can also include your own additional query parameters, for example, shopper ID or order reference number. If the URL to return to includes non-ASCII characters, like spaces or special letters, URL encode the value. The URL must not include personally identifiable information (PII), for example name or email address. |
riskData |
Device characteristics and other data that we use to detect fraudulent payment activity, and mitigate fraud. | |
applicationInfo
|
If you are building an Adyen solution for multiple merchants, include some basic identifying information, so that we can offer you better support. For more information, refer to Building Adyen solutions. | |
shopperEmail |
The shopper's email address. Strongly recommended because this field is used in a number of risk checks, and for 3D Secure. | |
shopperReference |
Your reference to uniquely identify this shopper. Minimum length: three characters. Do not include personally identifiable information, for example name or email address. Strongly recommended because this field is used in a number of risk checks. |
You need to include additional parameters in your payment request to:
- Integrate some payment methods. For more information, refer to our payment method integration guides.
- Make use of our risk management features. For more information, see Required risk fields.
- Use native 3D Secure 2 authentication.
- Tokenize your shopper's payment details or make recurring payments.
The following example shows how to make a payment request for EUR 10:
curl https://checkout-test.adyen.com/checkout/v71/payments \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d '{ "amount": { "currency": "EUR", "value": 1000 }, "reference": "YOUR_ORDER_NUMBER", "paymentMethod":{ "type": "scheme", "encryptedCardNumber": "test_4111111111111111", "encryptedExpiryMonth": "test_03", "encryptedExpiryYear": "test_2030", "encryptedSecurityCode": "test_737" }, "returnUrl": "https://your-company.com/checkout?shopperOrder=12xy..", "riskData": { "clientData": "eyJ0cmFuc1N0YXR1cy...I6IlkifQ==" }, "merchantAccount": "ADYEN_MERCHANT_ACCOUNT" }'
Next, implement logic to handle /payments responses with and without an action
object:
Response | Description | Next steps |
---|---|---|
No action object |
No additional steps are needed to complete the payment. | Get the payment outcome. |
With action object |
The shopper needs to do additional actions to complete the payment. | Pass the action and resultCode objects to the onSubmit actions.resolve() function. Drop-in handles the action accordingly. |
The following example shows the /payments response for iDEAL, a redirect payment method.
{ "resultCode": "RedirectShopper", "action": { "paymentMethodType": "ideal", "url": "https://checkoutshopper-test.adyen.com/checkoutshopper/checkoutPaymentRedirect?redirectData=X6Xtf...", "method": "GET", "type": "redirect" } }
Handle the additional action
Some payment methods require additional action from the shopper such as: to scan a QR code, to authenticate a payment with 3D Secure, or to log in to their bank's website to complete the payment.
Drop-in handles these additional front-end actions based on the action
object and resultCode
that you pass to the onSubmit
actions.resolve()
function.
The action.type
determines your next steps:
action.type |
Description | Next steps |
---|---|---|
voucher | Drop-in shows the voucher which the shopper uses to complete the payment. |
Get the payment outcome. |
redirect | Drop-in redirects the shopper to another website or app to complete the payment. | 1. When the shopper returns to your website, your server needs to handle the redirect result. 2. Send additional payment details to check the payment result. |
qrCode | Drop-in presents the QR code and calls the onAdditionalDetails event. |
1. Get the state.data from the onAdditionalDetails event and pass it your server. 2. Send additional payment details to check the payment result. |
await | Drop-in shows the waiting screen while the shopper completes the payment. Depending on the payment outcome, Drop-in calls the onAdditionalDetails or onError event. |
1. Get the state.data object from the onAdditionalDetails or onError event and pass it your server. 2. Send additional payment details to check the payment result. |
sdk | Drop-in presents the individual payment method's UI as an overlay and calls the onAdditionalDetails event. |
1. Get the state.data from the onAdditionalDetails event and pass it your server. 2. Send additional payment details to check the payment result. |
threeDS2 | The payment qualifies for 3D Secure 2, and will go through the authentication flow. Drop-in performs the authentication flow, and calls the onAdditionalDetails event. |
1. Get the state.data from the onAdditionalDetails event and pass it your server. 2. Send additional payment details to complete the payment. |
Handle the redirect result
If you receive an action.type
redirect, Drop-in redirects your shopper to another website to complete the payment.
After completing the payment, the shopper is redirected back to your returnUrl
with an HTTP GET. The returnUrl
is appended with a Base64-encoded redirectResult
:
GET /?shopperOrder=12xy..&&redirectResult=X6XtfGC3%21Y... HTTP/1.1 Host: www.your-company.com/checkout
- URL-decode the
redirectResult
and pass it to your server. - Send additional payment details.
If a shopper completed the payment but failed to return to your website, wait for the webhook to know the payment result.
Send additional payment details
If the shopper performed additional action, you need to submit additional payment details to either complete the payment, or to check the payment result.
Make a /payments/details request, and include:
- For
action.type
: redirect, pass in your request:details
: Object that contains the URL-decodedredirectResult
.
- For
action.type
: qrCode, await, sdk, or threeDS2, pass in your request thestate.data
from theonAdditionalDetails
event.
The following example shows how to submit additional payment details in case of a redirect.
curl https://checkout-test.adyen.com/checkout/v70/payments/details \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d '{ "details": { "redirectResult": "eyJ0cmFuc1N0YXR1cyI6IlkifQ==" } }'
The response includes:
pspReference
: Our unique identifier for the transaction.resultCode
: Indicates the status of the payment.
{ "pspReference": "NC6HT9CRT65ZGN82", "resultCode": "Authorised" }
{ "pspReference": "KHQC5N7G84BLNK43", "refusalReason": "Not enough balance", "resultCode": "Refused" }
Get the payment outcome
After Drop-in finishes the payment flow, you can show the shopper the current payment status. Adyen sends a webhook with the outcome of the payment.
Inform the shopper
Use the
resultCode
from the onPaymentCompleted
or onPaymentFailed
event to show the shopper the current payment status. This synchronous response doesn't give you the final outcome of the payment. You get the final payment status in a webhook that you use to update your order management system.
Update your order management system
You get the outcome of each payment asynchronously, in an AUTHORISATION webhook. Use the merchantReference
from the webhook to match it to your order reference.
For a successful payment, the event contains success
: true.
{ "live": "false", "notificationItems":[ { "NotificationRequestItem":{ "eventCode":"AUTHORISATION", "merchantAccountCode":"YOUR_MERCHANT_ACCOUNT", "reason":"033899:1111:03/2030", "amount":{ "currency":"EUR", "value":2500 }, "operations":["CANCEL","CAPTURE","REFUND"], "success":"true", "paymentMethod":"mc", "additionalData":{ "expiryDate":"03/2030", "authCode":"033899", "cardBin":"411111", "cardSummary":"1111" }, "merchantReference":"YOUR_REFERENCE", "pspReference":"NC6HT9CRT65ZGN82", "eventDate":"2021-09-13T14:10:22+02:00" } } ] }
For an unsuccessful payment, you get success
: false, and the reason
field has details about why the payment was unsuccessful.
{ "live": "false", "notificationItems":[ { "NotificationRequestItem":{ "eventCode":"AUTHORISATION", "merchantAccountCode":"YOUR_MERCHANT_ACCOUNT", "reason":"validation 101 Invalid card number", "amount":{ "currency":"EUR", "value":2500 }, "success":"false", "paymentMethod":"unknowncard", "additionalData":{ "expiryDate":"03/2030", "cardBin":"411111", "cardSummary":"1112" }, "merchantReference":"YOUR_REFERENCE", "pspReference":"KHQC5N7G84BLNK43", "eventDate":"2021-09-13T14:14:05+02:00" } } ] }
Error handling
In case you encounter errors in your integration, refer to the following:
- API error codes: If you receive a non-HTTP 200 response, use the
errorCode
to troubleshoot and modify your request. - Payment refusals: If you receive an HTTP 200 response with an Error or Refused
resultCode
, check the refusal reason and, if possible, modify your request.
Test and go live
Before going live, use our list of test cards and other payment methods to test your integration. We recommend testing each payment method that you intend to offer to your shoppers.
You can check the status of a test payment in your Customer Area, under Transactions > Payments.
To debug or troubleshoot test payments, you can also use API logs in your test environment.
When you are ready to go live, you need to:
- Apply for a live account. Review the process to start accepting payments on Get started with Adyen.
- Assess your PCI DSS compliance by submitting the Self-Assessment Questionnaire-A.
- Configure your live account.
- Submit a request to add payment methods in your live Customer Area .
- Switch from test to our live endpoints.
-
Load Drop-in from one of our live environments and set the
environment
to match your live endpoints:Endpoint region Value Europe (EU) live live United States (US) live live-us Australia (AU) live live-au Asia Pacific & Southeast (APSE) live live-apse India (IN) live live-in
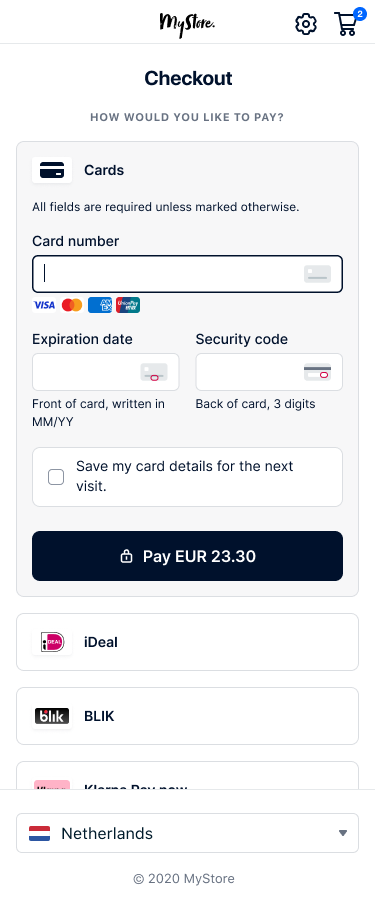
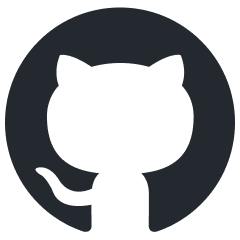
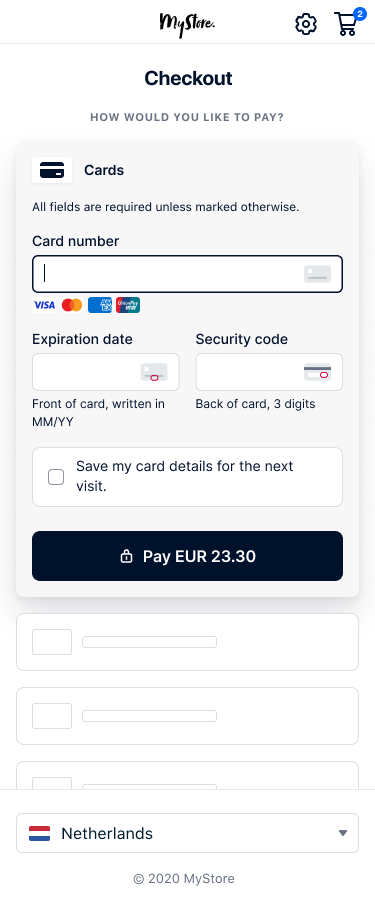
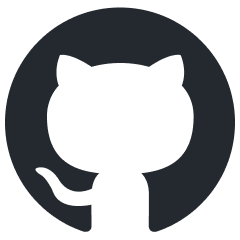
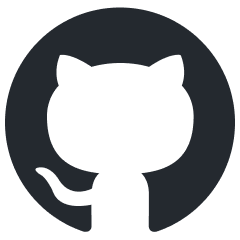
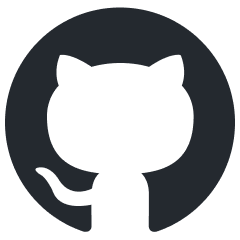
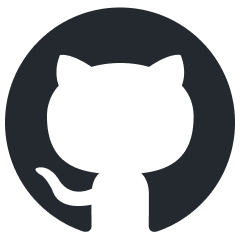
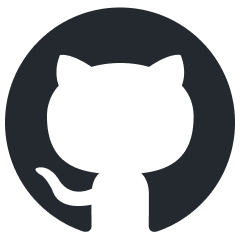
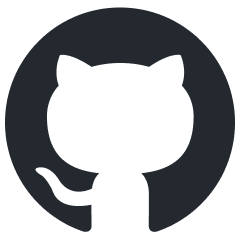
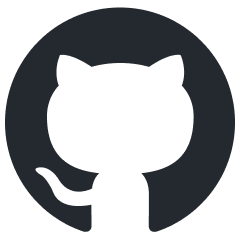
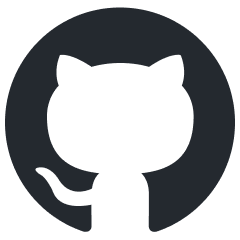
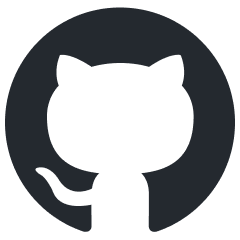