Flutter Drop-in
Render a list of available payment methods anywhere in your app.
Supported payment methods
Cards, buy now pay later, wallets, and many more.
See all supported payment methods
Features
- Low development time to integrate payment methods
- UI styling customization for the list of payment methods
- Adding payment methods to the list requires no extra development time
- 3D Secure 2 support built in
Start integrating with Flutter Drop-in
Choose your version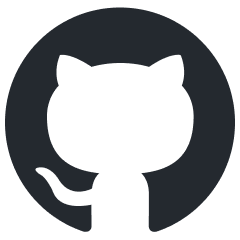
Adyen Flutter on GitHub
View the Adyen Flutter repository
View our example integrations
Drop-in is our pre-built UI solution for accepting payments in your app. Drop-in shows all payment methods as a list, in the same block. Your server makes one API request to the /sessions endpoint.
Requirements
Before you begin to integrate, make sure you have followed the Get started with Adyen guide to:
- Get an overview of the steps needed to accept live payments.
- Create your test account.
After you have created your test account:
- Get your API key.
- Get your client key.
- Set up webhooks to know the payment outcome.
Required versions:
- iOS 12 or later.
- Android 5.0 or later.
- Kotlin 1.8 or later.
- Gradle v8 or later.
How it works
For a Drop-in integration, you must implement the following parts:
- Your payment server: sends the API request to create a payment session.
- Your client app: shows the Drop-in UI where the shopper makes the payment. Drop-in uses the data from the API responses to handle the payment flow and additional actions on your client app.
- Your webhook server: receives webhooks that include the outcome of each payment.
The parts of your integration work together to complete the payment flow:
- The shopper goes to the checkout page.
- Your server uses the shopper's country and currency information from your client to create a payment session.
- Your client creates an instance of Drop-in using the session data from the server.
- Drop-in shows the available payment methods, collects the shopper's payment details, handles additional actions, and presents the payment result to the shopper.
- Your webhook server receives the notification containing the payment outcome.
If you are integrating these parts separately, you can start at the corresponding part of this integration guide:
Install an API library
We provide server-side API libraries for several programming languages, available through common package managers, like Gradle and npm, for easier installation and version management. Our API libraries will save you development time, because they:
- Use an API version that is up to date.
- Have generated models to help you construct requests.
- Send the request to Adyen using their built-in HTTP client, so you do not have to create your own.
Try our example integration
Requirements
- Java 11 or later.
Installation
You can use Maven, adding this dependency to your project's POM.
<dependency> <groupId>com.adyen</groupId> <artifactId>adyen-java-api-library</artifactId> <version>LATEST_VERSION</version> </dependency>
You can find the latest version on GitHub. Alternatively, you can download the release on GitHub.
Setting up the client
Create a singleton resource that you use for the API requests to Adyen:
// Import the required classes. package com.adyen.service; import com.adyen.Client; import com.adyen.service.checkout.PaymentsApi; import com.adyen.model.checkout.Amount; import com.adyen.model.checkout.CreateCheckoutSessionRequest; import com.adyen.model.checkout.CreateCheckoutSessionResponse; import com.adyen.enums.Environment; import com.adyen.service.exception.ApiException; import java.io.IOException; public class Snippet { public Snippet() throws IOException, ApiException { // Set up the client and service. Client client = new Client("ADYEN_API_KEY", Environment.TEST); } }
Create a payment session
A payment session is a resource with information about a payment flow initiated by the shopper. This resource has all the information required to handle all the stages of a payment flow. You can configure this resource with information like available payment methods, payment amount, or line items.
To create a payment session, make a POST /sessions request, including:
Parameter name | Required | Description |
---|---|---|
merchantAccount |
![]() |
Your merchant account name. |
amount |
![]() |
The currency and value of the payment, in minor units. This is used to filter the list of available payment methods to your shopper. |
returnUrl |
![]() |
The URL where the shopper should return after a redirection. For iOS, use the custom URL for your app, for example, my-app://adyen , to take the shopper back to your app. For more information on setting a custom URL scheme, read the Apple Developer documentation. You can also include your own additional query parameters, for example, shopper ID or order reference number. For Android, include your package name in the URL, for example, adyencheckout://your.package.name . Maximum length: 1024 characters. If the URL to return to includes non-ASCII characters, like spaces or special letters, URL encode the value. The URL must not include personally identifiable information (PII), for example name or email address. |
reference |
![]() |
Your unique reference for the payment. Minimum length: three characters. |
expiresAt |
The session expiry date in ISO8601 format, for example 2023-11-23T12:25:28Z, or 2023-05-27T20:25:28+08:00. When not specified, the expiry date is set to 1 hour after session creation. You cannot set the session expiry to more than 24 hours after session creation. | |
countryCode |
The shopper's country/region. This is used to filter the list of available payment methods to your shopper. Format: the two-letter ISO-3166-1 alpha-2 country code. Exception: QZ (Kosovo). |
|
channel |
The platform where the payment is taking place. Use iOS or Android. Strongly recommended because this field is used for 3D Secure. | |
shopperLocale |
The language that the payment methods will appear in. Set it to the shopper's language and country code. The default is en-US. Drop-in also uses this locale, if it is available. | |
shopperEmail |
The shopper's email address. Strongly recommended because this field is used in a number of risk checks, and for 3D Secure. | |
shopperReference |
Your reference to uniquely identify this shopper. Minimum length: three characters. Do not include personally identifiable information, for example name or email address. Strongly recommended because this field is used in a number of risk checks. | |
applicationInfo
|
If you are building an Adyen solution for multiple merchants, include some basic identifying information, so that we can offer you better support. For more information, refer to Building Adyen solutions. |
The following example shows how to create a session for a payment of 10 EUR:
curl https://checkout-test.adyen.com/checkout/v70/sessions \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d '{ "merchantAccount": "YOUR_MERCHANT_ACCOUNT", "amount": { "value": 1000, "currency": "EUR" }, "returnUrl": "my-app://adyen", "reference": "YOUR_PAYMENT_REFERENCE", "countryCode": "NL" }'
The response contains:
sessionData
: the payment session data.id
: a unique identifier for the session data.- The request body.
Pass the response to your client app, putting it in the sessionResponse
object.
{ "amount": { "currency": "EUR", "value": 1000 }, "countryCode": "NL", "expiresAt": "2021-08-24T13:35:16+02:00", "id": "CSD9CAC34EBAE225DD", "merchantAccount": "YOUR_MERCHANT_ACCOUNT", "reference": "YOUR_PAYMENT_REFERENCE", "returnUrl": "my-app://adyen", "sessionData": "Ab02b4c.." }
API error handling
If you do not get an HTTP 201 response, use the errorCode
field and the list of API error codes to troubleshoot.
Add Drop-in to your app
Add different configurations for iOS and Android, 'Drop-in', and some payment methods.
1. Add platform-specific configurations
-
Add the return URL handler to your
AppDelegate.swift
file:Add return URL handlerExpand viewCopy link to code blockCopy codeoverride func application(_: UIApplication, open url: URL, options _: [UIApplication.OpenURLOptionsKey: Any] = [:]) -> Bool { RedirectComponent.applicationDidOpen(from: url) return true } -
In your app, add a custom URL scheme that matches the
returnUrl
you use when creating the payment session. -
For voucher payment methods, add photo library usage descriptions to the
Info.plist
file. -
For Apple Pay, do the following:
- Complete the steps to set up Apple Pay with your own certificate.
- In your Runner target, add Apple Pay as a capability.
- In your Runner target, enter your merchant ID.
2. Create a configuration object
Create a configuration object with the following properties:
Parameter | Required | Description |
---|---|---|
environment |
![]() |
Use test. When you are ready to accept live payments, change the value to one of our live environments. |
clientKey |
![]() |
A public key linked to your API credential, used for client-side authentication. |
countryCode |
![]() |
The shopper's country/region. This is used to filter the list of available payment methods to your shopper. Format: the two-letter ISO-3166-1 alpha-2 country code. Exception: QZ (Kosovo). |
shopperLocale |
By default, this is set to en-US. To change the language, set this to the shopper's language and country code. If you included shopperLocale in your /sessions request, Drop-in uses that one. |
|
amount |
The currency and value of the payment, in minor units. This is used to filter the list of available payment methods to your shopper. |
For example:
final DropInConfiguration dropInConfiguration = DropInConfiguration( // Change the environment to live to accept live payments. environment: Environment.test, clientKey: CLIENT_KEY, countryCode: COUNTRY_CODE, shopperLocale: SHOPPER_LOCALE, // Optional. amount: AMOUNT, // Optional. // Configuration for specific payment methods. For example, Apple Pay. applePayConfiguration: applePayConfiguration );
To add configuration for specific payment methods, create an additional configuration object. For example, for Apple Pay:
final ApplePayConfiguration applePayConfiguration = ApplePayConfiguration( merchantId: MERCHANT_ID, merchantName: MERCHANT_NAME, );
Optional configuration
Parameter name | Description |
---|---|
preselectedPaymentMethodTitle Only for iOS. |
When you have a preselected payment method, sets the title of the Drop-in overlay. |
paymentMethodNames |
If you want to use custom names when displaying payment methods, set the name to use for each payment method. |
final DropInConfiguration dropInConfiguration = DropInConfiguration( // Change the environment to live to accept live payments. environment: Environment.test, clientKey: CLIENT_KEY, countryCode: COUNTRY_CODE, shopperLocale: SHOPPER_LOCALE, // Optional. amount: AMOUNT, // Optional. // Configuration for specific payment methods. For example, Apple Pay. applePayConfiguration: applePayConfiguration // Optional Drop-in configuration. paymentMethodNames: { "scheme": "Credit Card", }, );
3. Initialize Drop-in
Call the create
method, passing the following:
Parameter | Required | Description |
---|---|---|
sessionId |
![]() |
sessionResponse.id : the id from the /sessions response. |
sessionData |
![]() |
sessionData : the sessionData from the /sessions response. |
configuration |
![]() |
The configuration object you created. |
final SessionCheckout sessionCheckout = await AdyenCheckout.session.create( sessionId: sessionResponse.id, sessionData: sessionResponse.sessionData, configuration: dropInConfiguration, );
4. Show Drop-in in your app
Call startDropin
to show the Drop-in, passing the following:
Parameter | Required | Description |
---|---|---|
dropInConfiguration |
![]() |
The configuration object you created. |
checkout |
![]() |
The sessionCheckout you created. |
final PaymentResult paymentResult = await AdyenCheckout.session.startDropIn( dropInConfiguration: dropInConfiguration, checkout: sessionCheckout, );
Drop-in handles the payment flow.
5. Handle the result
When the payment flow is completed, you get one of the following objects:
Object | Description |
---|---|
PaymentSessionFinished |
The payment was successfully submitted. Contains a resultCode with the status of the payment. |
PaymentCancelledByUser |
The payment was canceled by the user. |
PaymentError |
The payment encountered an error. |
Handle the object and inform the shopper.
Get the payment outcome
After Drop-in finishes the payment flow, you can show the shopper the current payment status. Adyen sends a webhook with the outcome of the payment.
Inform the shopper
Use the
resultCode
to show the shopper the current payment status. This synchronous response doesn't give you the final outcome of the payment. You get the final payment status in a webhook that you use to update your order management system.
You can also get the result of the payment session on your server.
- Get the
id
from the /sessions response. - Get
sessionResult
from theonPaymentCompleted
event. -
Make a GET
/sessions/{id}?sessionResult={sessionResult}
request including theid
andsessionResult
. For example:Request for result of payment sessionExpand viewCopy link to code blockCopy codecurl -X GET https://checkout-test.adyen.com/checkout/v71/sessions/CS12345678?sessionResult=SOME_DATA
The response includes the result of the payment session (
status
). For example:Response with result of the payment sessionExpand viewCopy link to code blockCopy code{ "id": "CS12345678", "status": "completed" } Possible statuses:
status
Description completed The shopper completed the payment. This means that the payment was authorized. paymentPending The shopper is in the process of making the payment. This applies to payment methods with an asynchronous flow. canceled The shopper canceled the payment. expired The session expired (default: 1 hour after session creation). Shoppers can no longer complete the payment with this sessionId
.
The status
included in the response doesn't get updated. Do not make the request again to check for payment status updates. Instead, check webhooks or the Transactions list in your Customer Area.
Update your order management system
You get the outcome of each payment asynchronously, in an AUTHORISATION webhook. Use the merchantReference
from the webhook to match it to your order reference.
For a successful payment, the event contains success
: true.
{ "live": "false", "notificationItems":[ { "NotificationRequestItem":{ "eventCode":"AUTHORISATION", "merchantAccountCode":"YOUR_MERCHANT_ACCOUNT", "reason":"033899:1111:03/2030", "amount":{ "currency":"EUR", "value":2500 }, "operations":["CANCEL","CAPTURE","REFUND"], "success":"true", "paymentMethod":"mc", "additionalData":{ "expiryDate":"03/2030", "authCode":"033899", "cardBin":"411111", "cardSummary":"1111", "checkoutSessionId":"CSF46729982237A879" }, "merchantReference":"YOUR_REFERENCE", "pspReference":"NC6HT9CRT65ZGN82", "eventDate":"2021-09-13T14:10:22+02:00" } } ] }
For an unsuccessful payment, you get success
: false, and the reason
field has details about why the payment was unsuccessful.
{ "live": "false", "notificationItems":[ { "NotificationRequestItem":{ "eventCode":"AUTHORISATION", "merchantAccountCode":"YOUR_MERCHANT_ACCOUNT", "reason":"validation 101 Invalid card number", "amount":{ "currency":"EUR", "value":2500 }, "success":"false", "paymentMethod":"unknowncard", "additionalData":{ "expiryDate":"03/2030", "cardBin":"411111", "cardSummary":"1112", "checkoutSessionId":"861631540104159H" }, "merchantReference":"YOUR_REFERENCE", "pspReference":"KHQC5N7G84BLNK43", "eventDate":"2021-09-13T14:14:05+02:00" } } ] }
Test and go live
Before going live, use our list of test cards and other payment methods to test your integration. We recommend testing each payment method that you intend to offer to your shoppers.
You can check the status of a test payment in your Customer Area, under Transactions > Payments.
To debug or troubleshoot test payments, you can also use API logs in your test environment.
When you are ready to go live, you need to:
- Apply for a live account. Review the process to start accepting payments on Get started with Adyen.
- Assess your PCI DSS compliance by submitting the Self-Assessment Questionnaire-A.
- Configure your live account.
- Submit a request to add payment methods in your live Customer Area .
- Switch from test to our live endpoints.
-
Load Drop-in from one of our live environments and set the
environment
to match your live endpoints:Endpoint region Value Europe (EU) live europe United States (US) live unitedStates Australia (AU) live australia Asia Pacific & Southeast (APSE) live apse India (IN) live india
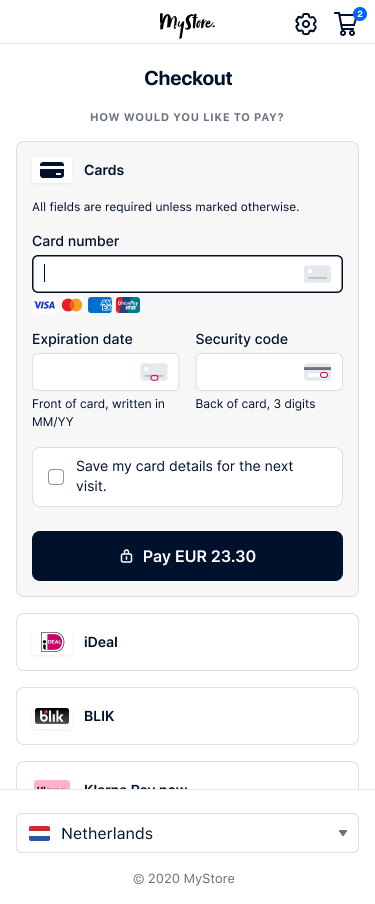
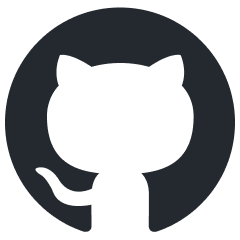
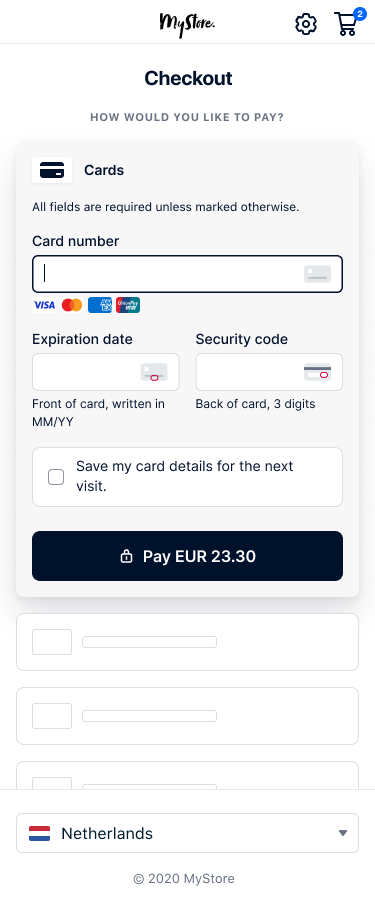
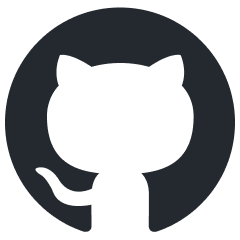
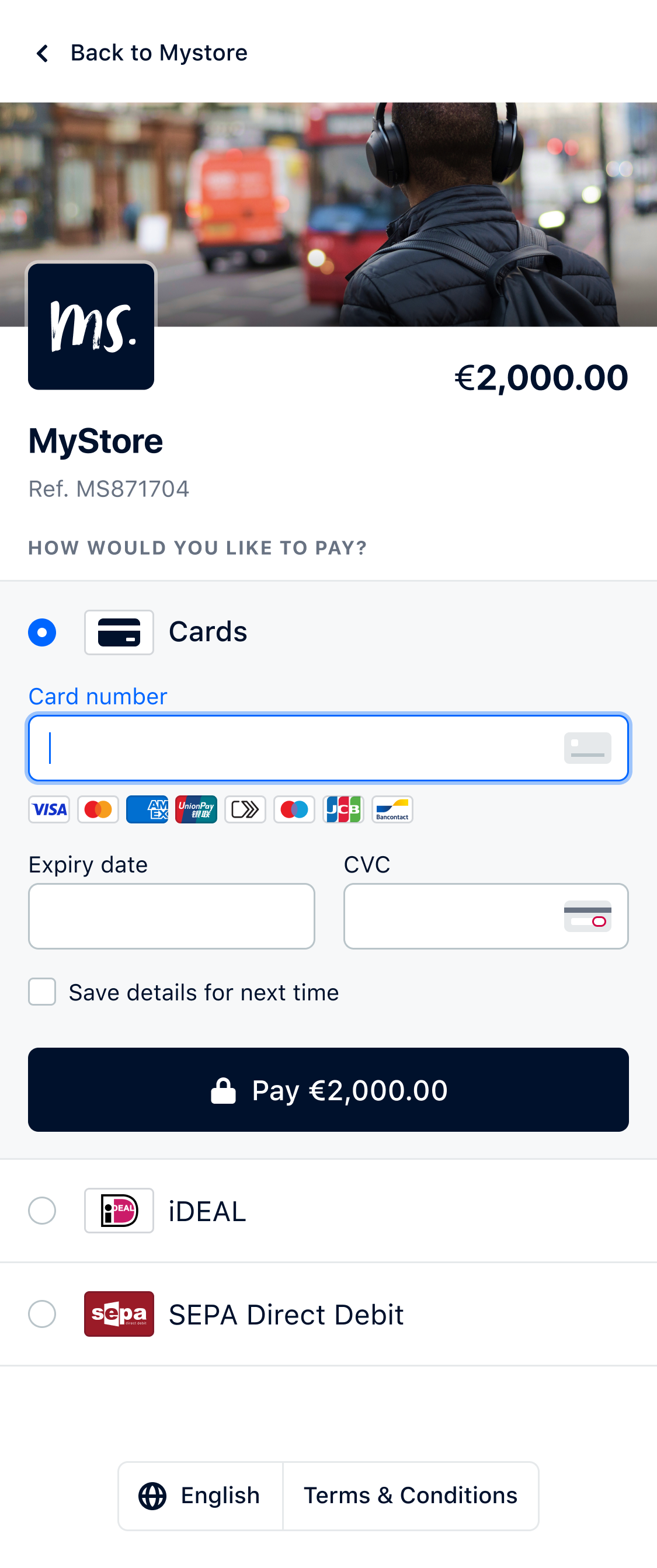
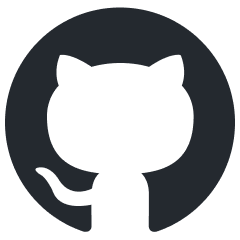
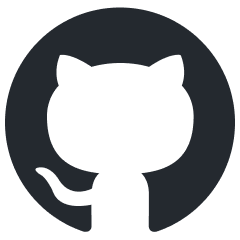
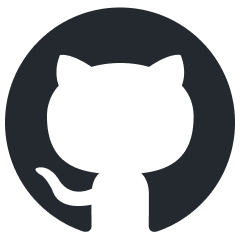
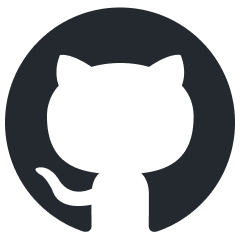
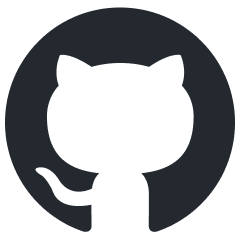
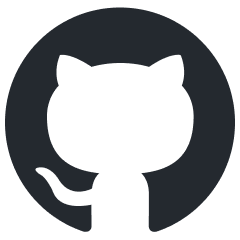
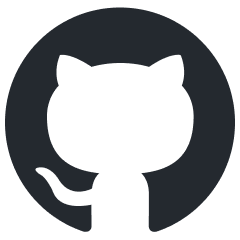