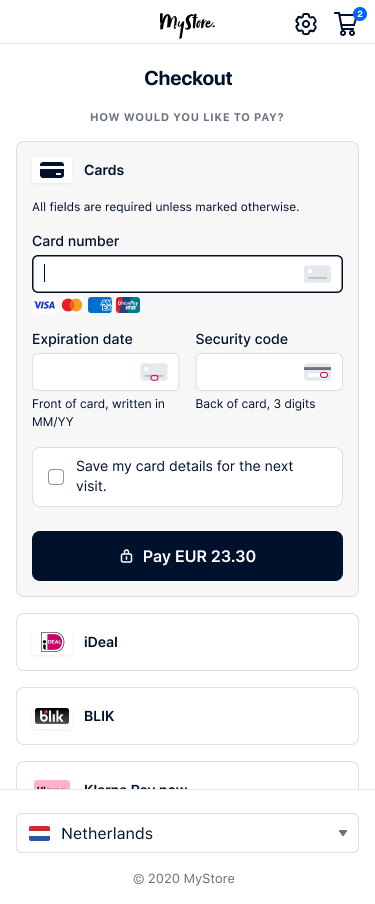
Web Drop-in
Render a list of available payment methods anywhere on your website.
Supported payment methods
Cards, buy now pay later, wallets, and many more.
See all supported payment methods
Features
- Lowest development time to integrate payment methods
- UI styling customization for the list of payment methods
- Adding payment methods to the list requires no extra development time
- 3D Secure 2 support built in
Demo
Start integrating with Web Drop-in
Choose your versionDrop-in is our pre-built UI solution for accepting payments on your website. Drop-in shows all payment methods as a list, in the same block. This Sessions flow integration requires you to make one API request to /sessions endpoint.
Adding new payment methods usually doesn't require more development work. Drop-in supports cards, wallets, and most local payment methods.
How it works
For a Drop-in integration, you must implement the following parts:
- Your payment server: sends the API request to create a payment session.
- Your client website: shows the Drop-in UI where the shopper makes the payment. Drop-in uses the data from the API responses to handle the payment flow and additional actions on your client website.
- Your webhook server: receives webhooks that include the outcome of each payment.
Integration steps
To integrate Drop-in in your web application:
- Install an API library on your server.
- Create a session from your server.
- Install the Adyen Web library on your front end.
- Create a DOM element for Drop-in.
- Configure and create an instance of
AdyenCheckout
. - Configure and create an instance of Drop-in.
- Handle redirects.
- Show the payment status to your shopper.
- Update your order management system.
- Test your integration and go live.
Payment flow
The parts of your integration work together to complete the payment flow. The payment flow is the same for all payments:
- The shopper goes to the checkout page.
- Your server uses the shopper's country and currency information from your client to create a payment session.
- Your client creates an instance of Drop-in using the session data from the server.
- Drop-in shows the available payment methods, collects the shopper's payment details, handles additional actions, and presents the payment result to the shopper.
- Your webhook server receives the notification containing the payment outcome.
If you are integrating these parts separately, you can start at the corresponding part of this integration guide:
Requirements
Before you begin to integrate, make sure you have followed the Get started with Adyen guide to:
- Get an overview of the steps needed to accept live payments.
- Create your test account.
After you have created your test account:
- Get your API key.
- Get your client key.
- Set up webhooks to know the payment outcome.
Install an API library
We provide server-side API libraries for several programming languages, available through common package managers, like Gradle and npm, for easier installation and version management. Our API libraries will save you development time, because they:
- Use an API version that is up to date.
- Have generated models to help you construct requests.
- Send the request to Adyen using their built-in HTTP client, so you do not have to create your own.
Try our example integration
Requirements
- Java 11 or later.
Installation
You can use Maven, adding this dependency to your project's POM.
<dependency> <groupId>com.adyen</groupId> <artifactId>adyen-java-api-library</artifactId> <version>LATEST_VERSION</version> </dependency>
You can find the latest version on GitHub. Alternatively, you can download the release on GitHub.
Setting up the client
Create a singleton resource that you use for the API requests to Adyen:
// Import the required classes. package com.adyen.service; import com.adyen.Client; import com.adyen.service.checkout.PaymentsApi; import com.adyen.model.checkout.Amount; import com.adyen.model.checkout.CreateCheckoutSessionRequest; import com.adyen.model.checkout.CreateCheckoutSessionResponse; import com.adyen.enums.Environment; import com.adyen.service.exception.ApiException; import java.io.IOException; public class Snippet { public Snippet() throws IOException, ApiException { // Set up the client and service. Client client = new Client("ADYEN_API_KEY", Environment.TEST); } }
Create a session
A payment session is a resource with information about a payment flow initiated by the shopper. This resource has all the information required to handle all the stages of a payment flow. You can configure this resource with information like available payment methods, payment amount, or line items.
To create a payment session, make a /sessions request, including:
Parameter name | Required | Description |
---|---|---|
merchantAccount |
![]() |
Your merchant account name. |
amount |
![]() |
The currency and value of the payment, in minor units. This is used to filter the list of available payment methods to your shopper. |
returnUrl |
![]() |
URL to where the shopper should be taken back to after a redirection. If the URL to return to includes non-ASCII characters, like spaces or special letters, URL encode the value. The URL must not include personally identifiable information (PII), for example name or email address. |
reference |
![]() |
Your unique reference for the payment. Minimum length: three characters. |
channel |
The platform where the payment is taking place. Use Web. | |
expiresAt |
The session expiry date in ISO8601 format, for example 2023-11-23T12:25:28Z, or 2023-05-27T20:25:28+08:00. When not specified, the expiry date is set to 1 hour after session creation. You cannot set the session expiry to more than 24 hours after session creation. | |
countryCode |
The shopper's country/region. This is used to filter the list of available payment methods to your shopper. Format: the two-letter ISO-3166-1 alpha-2 country code. Exception: QZ (Kosovo). |
|
shopperLocale |
The language that the payment methods will appear in. Set it to the shopper's language and country code. The default is en-US. The front end also uses this locale if it is available. | |
shopperEmail |
The shopper's email address. Strongly recommended because this field is used in a number of risk checks, and for 3D Secure. | |
shopperReference |
Your reference to uniquely identify this shopper. Strongly recommended because this field is used in a number of risk checks. | |
applicationInfo
|
If you are building an Adyen solution for multiple merchants, include some basic identifying information, so that we can offer you better support. For more information, refer to Building Adyen solutions. |
Here is an example of how to create a session for a payment of 10 EUR:
curl https://checkout-test.adyen.com/checkout/v71/sessions \ -H 'x-api-key: ADYEN_API_KEY' \ -H "idempotency-key: YOUR_IDEMPOTENCY_KEY" \ -H 'content-type: application/json' \ -d '{ "merchantAccount": "YOUR_MERCHANT_ACCOUNT", "amount": { "value": 1000, "currency": "EUR" }, "returnUrl": "https://your-company.com/checkout?shopperOrder=12xy..", "reference": "YOUR_PAYMENT_REFERENCE", "countryCode": "NL" }'
The response contains:
sessionData
: the payment session data you need to pass to your front end.id
: a unique identifier for the session data.- The request body.
{ "amount": { "currency": "EUR", "value": 1000 }, "countryCode": "NL", "expiresAt": "2021-08-24T13:35:16+02:00", "id": "CSD9CAC34EBAE225DD", "merchantAccount": "YOUR_MERCHANT_ACCOUNT", "reference": "YOUR_PAYMENT_REFERENCE", "returnUrl": "https://your-company.com/checkout?shopperOrder=12xy..", "sessionData": "Ab02b4c.." }
API error handling
If you do not get an HTTP 201 response, use the errorCode
field and the list of API error codes to troubleshoot.
Prepare your front end
Use Drop-in to show the available payment methods, and to collect payment details from your shoppers.
Get Adyen Web
Use the Adyen Web npm package, or embed the Adyen Web script and stylesheet into your HTML file:
Install the Adyen Web Node package:
npm install @adyen/adyen-web --save
Import Adyen Web into your application. You can add your own styling by overriding the rules in the CSS file.
import { AdyenCheckout } from '@adyen/adyen-web'; import '@adyen/adyen-web/styles/adyen.css';
Create a DOM element for Drop-in
Create a DOM container element on your checkout page where you want Drop-in to be rendered and give it a descriptive id
. We strongly recommend that you do not put it in an iframe element, because it may cause issues.
<div id="dropin-container"></div>
If you are using JavaScript frameworks such as Vue or React, make sure that you use references instead of selectors and that you do not re-render the DOM element.
Create a configuration object
Create an object for the global configuration of your Drop-in integration. This section shows the required and recommended parameters.
You can also use optional configuration to add features and to customize the checkout flow for your shoppers.
Parameter name | Required | Description |
---|---|---|
session |
![]() |
The payment session object from your call to /sessions . Contains a session.id and session.sessionData . |
clientKey |
![]() |
A public key linked to your API credential, used for client-side authentication. |
environment |
![]() |
Use test. When you are ready to accept live payments, change the value to one of our live environments. |
onPaymentCompleted(result, component) |
![]() |
Create an event handler, called when the payment is completed. |
onError(error) |
Create an event handler, called when an error occurs in Drop-in. | |
paymentMethodsConfiguration |
Configuration for specific payment methods. The payment method guides have configuration options specific to each payment method. | |
analytics.enabled |
Indicates if you are sending analytics data to Adyen. Default: true. |
const configuration = { environment: 'test', // Change to 'live' for the live environment. clientKey: 'test_870be2...', // Public key used for client-side authentication: https://docs.adyen.com/development-resources/client-side-authentication analytics: { enabled: true // Set to false to not send analytics data to Adyen. }, session: { id: 'CSD9CAC3...', // Unique identifier for the payment session. sessionData: 'Ab02b4c...' // The payment session data. }, onPaymentCompleted: (result, component) => { console.info(result, component); }, onError: (error, component) => { console.error(error.name, error.message, error.stack, component); }, // Any payment method specific configuration. Find the configuration specific to each payment method: https://docs.adyen.com/payment-methods // For example, this is 3D Secure configuration for cards: paymentMethodsConfiguration: { card: { hasHolderName: true, holderNameRequired: true, billingAddressRequired: true } } };
Optional configuration
The dropin
instance only accepts parameters related to itself. You must set global or component-specific configuration either on the main instance,
AdyenCheckout
, or in the paymentMethodsConfiguration
object.
Any configuration on paymentMethodsConfiguration
overrides configuration set globally, on AdyenCheckout
.
const checkout = await AdyenCheckout({ onError: () => {}, // Global configuration for onError paymentMethodsConfiguration: { card: { onError: () => {}, // onError configuration for card payments. Overrides the global configuration. } } }); checkout.create('dropin', { onReady: () => {}, // Drop-in configuration only has props related to itself, like the onReady event. Drop-in configuration cannot contain generic configuration like the onError event. });
These parameters are specific to Drop-in and are only accepted on the DropIn
instance.
Parameter name | Description |
---|---|
openFirstPaymentMethod |
When enabled, Drop-in opens the first payment method automatically on page load. Defaults to true. |
openFirstStoredPaymentMethod |
When enabled, Drop-in opens the payment method with stored card details on page load. This option takes precedence over openFirstPaymentMethod . Defaults to true. |
showStoredPaymentMethods |
Shows or hides payment methods with stored card details. Defaults to true. |
showRemovePaymentMethodButton |
Allows the shopper to remove a stored payment method. Defaults to false. If using this prop, you must also implement the onDisableStoredPaymentMethod callback. |
showPaymentMethods |
Shows or hides regular (not stored) payment methods. Set to false if you only want to show payment methods with stored card details. Defaults to true. |
onReady() |
Called when Drop-in is initialized and is ready for use. |
onSelect(component) |
Called when the shopper selects a payment method. |
onDisableStoredPaymentMethod(storedPaymentMethodId, resolve, reject) |
Called when a shopper removes a stored payment method. To remove the selected payment method, make a /disable request using the storedPaymentMethodId . Then call either resolve() or reject() , depending on the /disable response. |
instantPaymentTypes |
Moves payment methods to the top of the list of available payment methods. This is available for Apple Pay and Google Pay. |
Properties
Parameter name | Description |
---|---|
amount |
Amount to be displayed on the Pay Button. It expects an object with the value and currency properties. For example, { value: 1000, currency: 'USD' } . |
showPayButton |
Shows or hides a Pay Button for each payment method. Defaults to true. When set to false, you must override it in paymentMethodsConfiguration
. The Pay button triggers the onSubmit event when payment details are valid. If you want to disable the button and then trigger the submit flow on your own, set this to false and call the .submit() method from your own button implementation. PayPal Smart Payment Buttons doesn't support the .submit() method. |
locale |
The language used in the Drop-in UI. For possible values, see the list of available languages. By default, this is the either the shopperLocale from your /sessions request or, if this locale is not available on Drop-in, en-US. |
setStatusAutomatically v4.7.0 or later |
Set to false to not set the Drop-in status to 'loading' when onSubmit is triggered. Defaults to true. Setting to false can result in your shoppers pressing the Pay button twice. |
secondaryAmount |
Shows the payment amount in an additional currency on the Pay button. You must do the currency conversion and set the amount. This object has: - currency : The three-character ISO currency code. - value : The amount of the transaction, in minor units. - currencyDisplay : Sets the currency formatting. Default: symbol. |
Methods
Method name | Description |
---|---|
mount(selector) |
Mounts the Drop-in into the DOM returned by the selector . The selector must be either a valid CSS selector string or an HTMLElement reference. |
unmount() |
Unmounts the Drop-in from the DOM. We recommend to unmount in case the payment amount changes after the initial mount. |
closeActivePaymentMethod() |
Closes a selected payment method, for example if you want to reset the Drop-in. |
update() |
Updates the properties and remounts Drop-in into the DOM, for example, if you want to change the properties of the configuration object after the Drop-in is mounted. |
Events
Event name | Description |
---|---|
beforeSubmit(data, component, actions) |
Create an event handler, called when the shopper selects the Pay button. Do not use this if you use additional methods to make additional API requests. Allows you to add parameters to the payment request that Drop-in makes. For example, you can add shopper details like billingAddress, deliveryAddress
,
shopperEmail
, or
shopperName
. You can do the following: - Continue the payment flow: add additional parameters like billingAddress , shopperName , shopperEmail , and deliveryAddress to the data object. Call actions.resolve() , passing data to it. Stop the payment flow, for example, if the product is out of stock: call actions.reject() . |
onError(error) |
Create an event handler, called when an error occurs in Drop-in . |
onChange(state, component) |
Create an event handler, called when a change happens in the payment form. |
onActionHandled |
Create an event handler, called when an action, for example a QR code or 3D Secure 2 authentication screen, is shown to the shopper. The following action.type values trigger this callback: - threeDS - qr - await Returns data that contains: - componentType : The type of component that shows the action to the shopper. - actionDescription : A description of the action shown to the shopper. |
onSubmit(state, component) |
This additional method is required if you want to update the payment amount after rendering Drop-in. Create an event handler, called when the shopper selects the Pay button and payment details are valid. Makes a /payments request. |
onAdditionalDetails(state, component) |
This additional method is required if you want to confirm an additional action on your server. Create an event handler, called when a payment method requires more details, for example for native 3D Secure 2, or native QR code payment methods. Makes a /payments/details request. |
Drop-in orders the payment methods by popularity, the most popular payment methods in the shopper's country appearing at the top.
In your Customer Area, you can configure which payment methods are rendered based on the shopper's country. If you want to customize rendered payment methods for specific transactions, you can do this on your server.
Customize based on shopper's country
To configure which payment methods are rendered (and in which order) based on the shopper's country:
-
Log in to your Customer Area.
-
Go to Settings > Checkout settings.
If Checkout settings does not appear in the Settings menu, ask your admin user to give you the Change payment methods user role.
-
Select a Shopper country.
-
Drag the payment methods into the order you want them to appear to shoppers in this country.
-
To hide a payment method from shoppers in this country, drag it to the Other configured payment methods box.
Customize for specific transactions (on your server)
When making a /sessions request, you can include one of the following parameters:
- allowedPaymentMethods: Drop-in renders only the payment methods that you specify.
- blockedPaymentMethods: Drop-in doesn't render payment methods that you specify.
To refer to payment methods, use their paymentMethod.type
from Payment methods overview.
The following example shows how to make a request to only show iDEAL and credit cards in the payment form:
curl https://checkout-test.adyen.com/checkout/v70/sessions \ -H 'x-api-key: ADYEN_API_KEY' \ -H 'content-type: application/json' \ -d '{ "merchantAccount": "YOUR_MERCHANT_ACCOUNT", "allowedPaymentMethods": ["ideal", "scheme"], "countryCode": "NL", "amount": { "currency": "EUR", "value": 1000 }, "channel": "Web", "shopperLocale": "NL" }'
The Drop-in UI can be styled to match your website and brand. The styling of fonts, colors, layouts, and buttons can be customized using CSS.
Using custom styling
Drop-in includes a pre-styled payment form. You can override this styling using Drop-in CSS classes and common CSS properties.
To override the default Drop-in styling:
-
In your CSS, add Drop-in classes that represent styling that you want to customize in your payment form.
-
Customize the styling of these classes using common CSS properties.
For example, to change the Pay button to have:
- Green background (
#0abf53
). - Heavier text.
- Square corners.
Include the following to your CSS:
Add button stylingExpand viewCopy link to code blockCopy code.adyen-checkout__button--pay { background-color: #0abf53; font-weight: 900; border-radius: 0; } - Green background (
We recommend testing that your styling renders correctly on multiple browsers, device types (desktop and mobile), and viewport sizes.
Drop-in CSS classes
You can customize the styling for Drop-in using the CSS classes below.
These classes follow the BEM (.block__element--modifier
) methodology, where state is represented by a modifier (such as --selected
).
Some payment methods have their own unique CSS classes that aren't shown in the sample below. For a full list of classes that you can style, inspect your instance of Drop-in using your browser's developer tools.
.adyen-checkout__payment-method { /* Payment method container */ } .adyen-checkout__payment-method--selected { /* Payment method that has been selected */ } .adyen-checkout__payment-method__header { /* Payment method icon and name */ } .adyen-checkout__payment-method__radio { /* Radio button in payment method header */ } .adyen-checkout__payment-method__radio--selected { /* Selected radio button in payment method header */ } .adyen-checkout__payment-method__name { /* Payment method name in the payment method header */ } .adyen-checkout__spinner__wrapper { /* Spinning icon */ } .adyen-checkout__button { /* Buttons */ } .adyen-checkout__button--pay { /* Pay button */ } .adyen-checkout__field { /* Form field container */ } .adyen-checkout__label { /* Form label container */ } .adyen-checkout__label__text { /* Text element inside the form label container */ } .adyen-checkout__input { /* Input fields */ } .adyen-checkout__input--error { /* Error state for the input fields */ } .adyen-checkout__error-text { /* Error message text */ } .adyen-checkout__card__cardNumber__input { /* Input field for the card number */ } .adyen-checkout__field--expiryDate { /* Input field for the expiry date */ } .adyen-checkout__field__cvc { /* Input field for the CVC security code */ } .adyen-checkout__card__holderName { /* Input field for cardholder name */ } .adyen-checkout__checkbox__input { /* Checkboxes */ } .adyen-checkout__checkbox__label { /* Checkbox labels */ } .adyen-checkout__radio_group__input { /* Radio buttons */ } .adyen-checkout__dropdown__button { /* Dropdown button showing list of options */ } .adyen-checkout__dropdown__list { /* Dropdown list */ } .adyen-checkout__dropdown__element { /* Elements in the dropdown list */ } .adyen-checkout__link { /* Links */ }
Styling card input fields
If you want to change the styling of the card number, CVC, and expiry date of a card, you can do so by including a
styles
property in the card
configuration when creating the main AdyenCheckout
instance.
You can provide styling for the following:
base
: Base styling applied to the iframe. All styling extends from this style.error
: Styling applied when a field fails validation.placeholder
: Styling applied to the field's placeholder values.validated
: Styling applied once a field passes validation.
For a list of supported properties, refer to Styling card input fields.
For example, to change the color of the card input fields to white:
const checkout = new AdyenCheckout({ paymentMethodsConfiguration: { card: { styles: { base: { color: "#ffffff" // CSS color code for white } } } } });
Supported languages
We include UI localizations for some languages. The fields and text are in the files for the included locales.
To use a language or localization that isn't included, create your own.
Change the language
By default, the UI is presented according to the shopperLocale
from your /sessions request. If this language isn't supported, the UI is presented in US English.
You can also set the language by specifying a locale
on the AdyenCheckout
instance.
Customize the localization
The text displayed in each localization can be customized, allowing you to replace the default text with your own.
To customize a localization:
-
Create a
translations
object on your payments page. -
In this object, specify the
locale
you want to customize, and add key-value pairs corresponding to any text you want to customize.The following example shows how to customize the US English (
en-US
) translation so that:- Shipping Address appears instead of Delivery Address (the default en-US text).
- State appears instead of State or Province (the default en-US text).
Customize translationsExpand viewCopy link to code blockCopy codeconst translations = { "en-US": { "deliveryAddress": "Shipping Address", "stateOrProvince": "State" } }; -
To use the customized localization in your payment form, provide the following parameters in the
configuration
object:Parameter name Required Description locale
The locale
you customized in the previous step.translations
Use translations The following example shows how to use a customized en-US localization in Drop-in.
Example of US English localizationExpand viewCopy link to code blockCopy codeconst configuration = { locale: "en-US", translations: translations, ... };
Create your custom localization
To use a language or localization that we do not included, create your own.
-
Create a
translations
object on your payments page. -
In this object, specify the
locale
you want to create. For example, you can use the locale en-GB to create a British English localization, and add key-value pairs corresponding to text shown in the UI.The example below shows a
translation
object for creating a en-GB localization.British English translations exampleExpand viewCopy link to code blockCopy codeconst translations = { "en-GB": { "paymentMethods.moreMethodsButton": "More payment methods", "payButton": "Pay", "storeDetails": "Save for my next payment", ... } }; -
To use your localization in your payment form, provide the following parameters in the
configuration
object:Parameter name Required Description locale
The locale
you created in the previous step.translations
Use translations The example below shows how to use a created localization for en-GB.
const configuration = { locale: "en-GB", translations: translations, ... }; const checkout = new AdyenCheckout(configuration);
Text direction
The default text direction is left-to-right. Text direction is independent from the locale
you set in the configuration
object. So even if you set the locale to a right-to-left language, like Arabic, you still need to set the text direction.
To change the text direction to right-to-left, use the HTML dir
attribute on the parent element for the checkout container.
Initialize the payment session
- Create an instance of
AdyenCheckout
using the configuration object you created. - Create an instance of Drop-in and mount it to the container element you created.
// Create an instance of AdyenCheckout using the configuration object. const checkout = await AdyenCheckout(configuration); // Create an instance of Drop-in and mount it to the container you created. const dropinComponent = checkout.create('dropin').mount('#dropin-container');
Handle the redirect
Some payment methods, like iDEAL and some 3D Secure flows, will redirect the shopper back to your website. When the shopper comes back to your website, show them the payment result, based on the result code. To get the resultCode
, you can either:
- Create an instance of
AdyenCheckout
after the redirect, as described below. - Confirm the redirect result on your server, for which you need to implement an extra API endpoint.
The shopper comes back to the returnUrl
specified when creating the payment session. The returnUrl
has query parameters appended to it, which you need to handle the redirect:
sessionId
: the unique identifier for the shopper's payment session.redirectResult
: details you need to submit to handle the redirect.
If the shopper doesn't return to you website, you do not get a redirectResult
. You do not need to do anything to handle the redirect in this case. Instead, wait for the webhook that we send to your server.
// The return URL has query parameters related to the payment session. https://your-company.com/?sessionId=CSD9CAC34EBAE225DD&redirectResult=X6XtfGC3!Y...
Extract the values from the query string parameters and create a function which handles the redirect result. The function needs to:
- Create an instance of Adyen Checkout using the
sessionId
value you extracted. - Submit the
redirectResult
value you extracted from thereturnUrl
.
// Create an instance of AdyenCheckout to handle the shopper returning to your website. // Configure the instance with the sessionId you extracted from the returnUrl. const checkout = await AdyenCheckout(configuration); // Submit the redirectResult value you extracted from the returnUrl. checkout.submitDetails({ details: { redirectResult: redirectResult } });
If the shopper doesn't return to your website, do not call submitDetails
, because the result doesn't change when you attempt the request.
After you submit the redirectResult
value, Drop-in calls the onPaymentCompleted(result, component)
event. Use the result code in result.resultCode
to inform the shopper.
To update your order management system, wait for the webhook that we send to your server.
Handle errors
If an error occurs, the onError
event returns an object which contains details about the error:
Error field | Description |
---|---|
error.name |
The type of error. Use the values it returns to configure localized error messages for your shoppers:
|
error.message |
Gives more information for each type of error. The message is technical so you shouldn't show it to your shoppers. For error.name : NETWORK_ERROR, the information in the message field depends on the environment:
|
component |
The name of the variable where you created the instance of Drop-in, for example dropinComponent. |
The error
object may contain additional fields inherited from the Error()
constructor.
{ onError: (error, component) => { console.error(error.name, error.message, component); } }
Get the payment outcome
After Drop-in finishes the payment flow, you can show the shopper the current payment status. Adyen sends a webhook with the outcome of the payment.
Inform the shopper
From the onPaymentCompleted
event, you can get the
resultCode
to inform the shopper about the current payment status.
You can also get the result of the payment session on your server.
- Get the
id
from the/sessions
response. - Get
sessionResult
from theonPaymentCompleted
event. -
Make a GET
/sessions/{id}?sessionResult={sessionResult}
request including theid
andsessionResult
. For example:Request for result of payment sessionExpand viewCopy link to code blockCopy codecurl -X GET https://checkout-test.adyen.com/checkout/v70/sessions/CS12345678?sessionResult=SOME_DATA
The response includes the result of the payment session (
status
). For example:Response with result of the payment sessionExpand viewCopy link to code blockCopy code{ "id": "CS12345678", "status": "completed" } Possible statuses:
status
Description completed The shopper completed the payment. This means that the payment was authorized. paymentPending The shopper is in the process of making the payment. This applies to payment methods with an asynchronous flow. canceled The shopper canceled the payment. expired The session expired (default: 1 hour after session creation). Shoppers can no longer complete the payment with this sessionId
.
The status
included in the response doesn't get updated. Do not make the request again to check for payment status updates. Instead, check webhooks or the Transactions list in your Customer Area.
Update your order management system
You get the outcome of each payment asynchronously, in an AUTHORISATION webhook. Use the merchantReference
from the webhook to match it to your order reference.
For a successful payment, the event contains success
: true.
{ "live": "false", "notificationItems":[ { "NotificationRequestItem":{ "eventCode":"AUTHORISATION", "merchantAccountCode":"YOUR_MERCHANT_ACCOUNT", "reason":"033899:1111:03/2030", "amount":{ "currency":"EUR", "value":2500 }, "operations":["CANCEL","CAPTURE","REFUND"], "success":"true", "paymentMethod":"mc", "additionalData":{ "expiryDate":"03/2030", "authCode":"033899", "cardBin":"411111", "cardSummary":"1111", "checkoutSessionId":"CSF46729982237A879" }, "merchantReference":"YOUR_REFERENCE", "pspReference":"NC6HT9CRT65ZGN82", "eventDate":"2021-09-13T14:10:22+02:00" } } ] }
For an unsuccessful payment, you get success
: false, and the reason
field has details about why the payment was unsuccessful.
{ "live": "false", "notificationItems":[ { "NotificationRequestItem":{ "eventCode":"AUTHORISATION", "merchantAccountCode":"YOUR_MERCHANT_ACCOUNT", "reason":"validation 101 Invalid card number", "amount":{ "currency":"EUR", "value":2500 }, "success":"false", "paymentMethod":"unknowncard", "additionalData":{ "expiryDate":"03/2030", "cardBin":"411111", "cardSummary":"1112", "checkoutSessionId":"861631540104159H" }, "merchantReference":"YOUR_REFERENCE", "pspReference":"KHQC5N7G84BLNK43", "eventDate":"2021-09-13T14:14:05+02:00" } } ] }
Test and go live
Before going live, use our list of test cards and other payment methods to test your integration. We recommend testing each payment method that you intend to offer to your shoppers.
You can check the status of a test payment in your Customer Area, under Transactions > Payments.
To debug or troubleshoot test payments, you can also use API logs in your test environment.
When you are ready to go live, you need to:
- Apply for a live account. Review the process to start accepting payments on Get started with Adyen.
- Assess your PCI DSS compliance by submitting the Self-Assessment Questionnaire-A.
- Configure your live account.
- Submit a request to add payment methods in your live Customer Area .
- Switch from test to our live endpoints.
-
Load Drop-in from one of our live environments and set the
environment
to match your live endpoints:Endpoint region Value Europe (EU) live live United States (US) live live-us Australia (AU) live live-au Asia Pacific & Southeast (APSE) live live-apse India (IN) live live-in
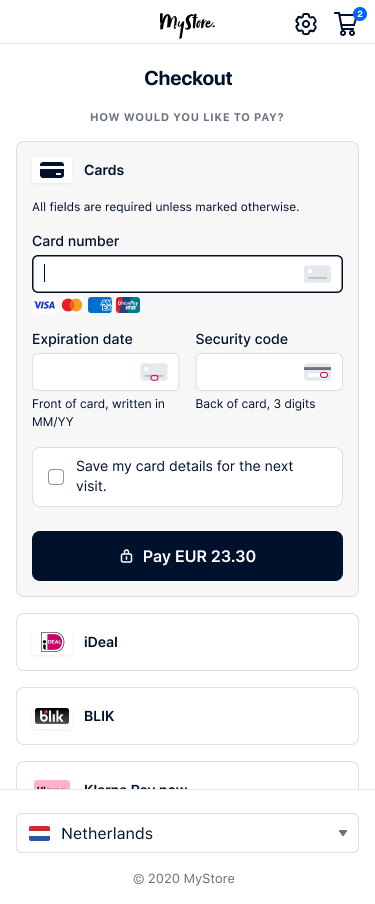
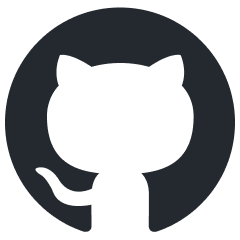
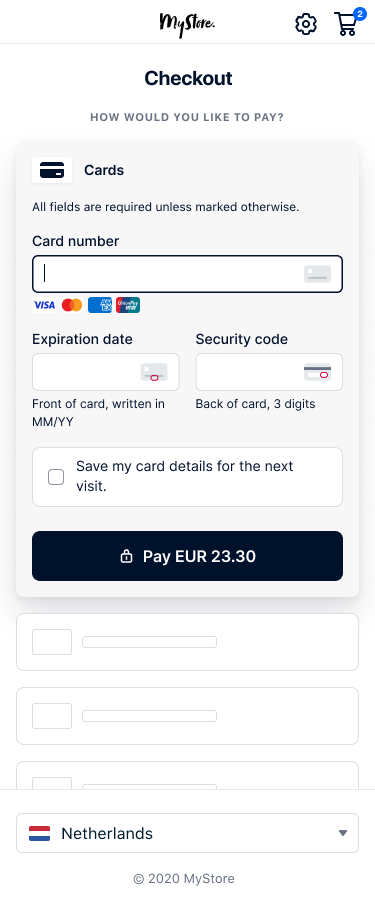
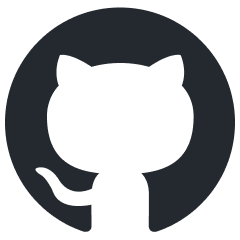
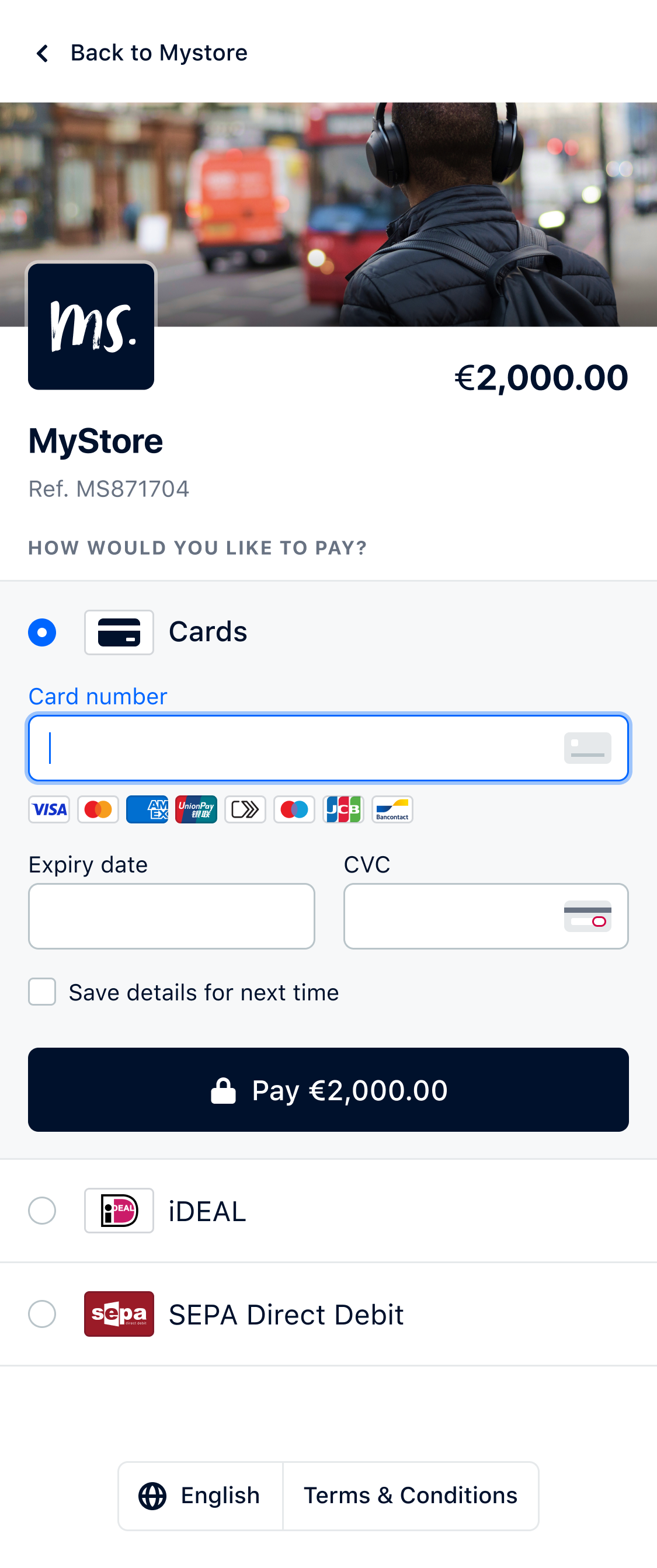
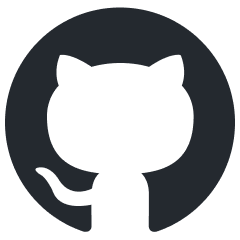
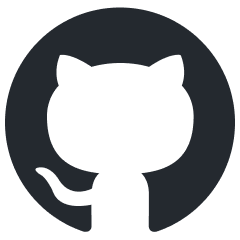
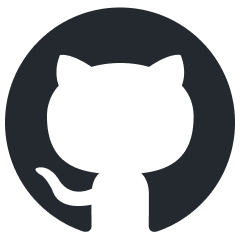
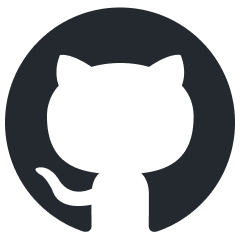
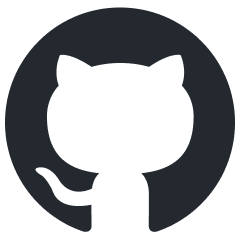
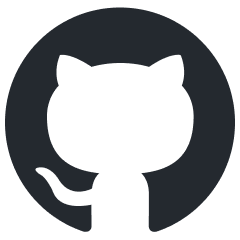
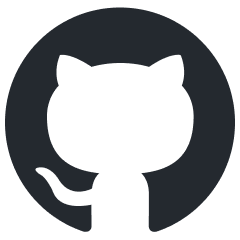