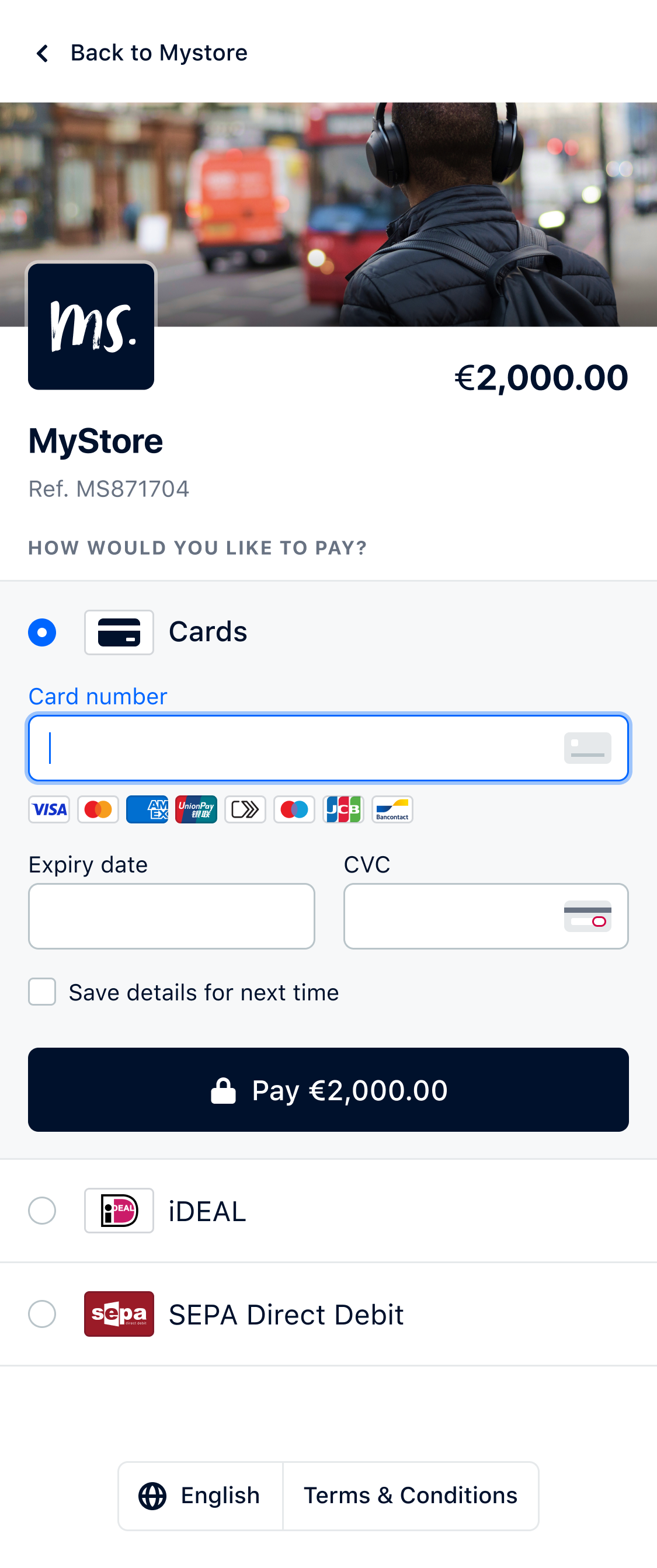
Start integrating with Web Hosted Checkout
For more features in addition to making payments and more styling options, we recommend integrating with our Drop-in or Components instead.
Feature | Hosted Checkout | Drop-in/Components |
---|---|---|
Make a payment with any available payment method. | ![]() |
![]() |
Customize styling elements, for example button color and shape, of individual payment methods. | ![]() |
|
Update the payment amount after starting the payment session. | ![]() |
|
Apple Pay payments with your own certificate. | ![]() |
- ACH Direct Debit
- Affirm
- Afterpay (AU, NZ, US, CA)
- Alipay
- AlipayHK
- Apple Pay
- Atome
- BACS Direct Debit
- Bancontact Card
- Bancontact Mobile (Payconiq)
- Benefit
- BLIK
- Boleto Bancário
- Cards, including 3D Secure 1 and 3D Secure 2 authentication
- Clearpay
- Credit card installments
- Stored card details
- DANA
- Dragonpay
- EPS
- Fawry
- GCash
- Gift cards
- Google Pay
- GrabPay
- iDEAL
- Indonesian bank transfers and convenience stores
- Japanese convenience stores (Konbini)/
7-Eleven Japan - KakaoPay
- Klarna
- KNET
- MB WAY
- MobilePay
- MOLPay
- MoMo
- Multibanco
- Oney 3x4x
- Online banking Finland
- Online banking India
- Online banking Japan
- Online banking Poland
- Napas card
- OXXO
- PayBright
- PayPal
- Paytm
- PayU
- Pix
- Ratepay
- SEPA Direct Debit
- Sofort
- Swish
- Trustly
- TWINT
- Vipps
- Wallets India
- WeChat Pay
- Zip
How it works
When the shopper goes to checkout on your website, you redirect them to a Hosted Checkout page. After they make the payment, the shopper gets redirected to your page, and you show the shopper the outcome of the payment session.
You make two API requests to the /sessions endpoint:
- When the shopper goes to checkout, make a request to get the URL to the Hosted Checkout page.
- After the shopper pays, make a request to get the outcome of the payment session.
Requirements
Before you begin to integrate, make sure you have followed the Get started with Adyen guide to:
- Get an overview of the steps needed to accept live payments.
- Create your test account.
After you have created your test account:
- Get your API key.
- Get your client key.
- Set up webhooks to know the payment outcome.
Install an API library
Try our example integration
Requirements
- Ruby 2.7 or later.
Installation
You can use RubyGems:
gem install adyen-ruby-api-library
Alternatively, you can download the release on GitHub.
Run bundle install
to install dependencies.
Configure your theme
To create a theme, you must have one of the following user roles:
- Merchant admin
- Hosted Checkout and Pay by Link Settings
To create a new theme:
- Log in to your Customer Area and switch to your merchant account if necessary.
- Go to Pay by Link > Themes.
- Select Create a new theme.
- Enter a Theme name. This name helps you to identify different themes.
- Enter a Display name. This name is visible to the shopper on the Hosted Checkout page.
- Upload a brand logo.
- Upload a background image.
- Enter a background color. This color is used instead of the background image if the shopper's internet connection is too slow.
- If you want this to be the default theme for all Hosted Checkout pages, select Set as default. Available only on the merchant account.
- Select Create.
Get the theme ID:
- Go to Pay by Link > Themes.
- Select the options icon from the theme.
- Select Copy theme ID.
This copies the theme ID to your system's clipboard.
Create a payment session
When the shopper goes to checkout, for example by selecting a Checkout button, make a POST /sessions request from your server, including:
Parameter | Required | Description |
---|---|---|
amount |
![]() |
The currency and value of the payment, in minor units. This is used to filter the list of available payment methods to your shopper. |
merchantAccount |
![]() |
Your merchant account name. |
mode |
![]() |
hosted |
themeId |
![]() |
The theme ID of the theme to use for the Hosted Checkout page. |
returnUrl |
![]() |
URL where to redirect the shopper after they make the payment on the Hosted Checkout page. The URL can contain a maximum of 1024 characters and should include the protocol: http:// or https:// . You can also include your own additional query parameters, for example, shopper ID or order reference number. If the URL to return to includes non-ASCII characters, like spaces or special letters, URL encode the value. The URL must not include personally identifiable information (PII), for example name or email address. |
reference |
![]() |
Your unique reference for the payment. Minimum length: three characters. |
countryCode |
The shopper's country/region. This is used to filter the list of available payment methods to your shopper. Format: the two-letter ISO-3166-1 alpha-2 country code. Exception: QZ (Kosovo). |
|
expiresAt |
The expiration time and date of the Hosted Checkout page, in ISO8601 format. The default is 1 hour after creation. You cannot set this to more than 24 hours after creation. |
For example:
curl https://checkout-test.adyen.com/checkout/v70/sessions \ -H 'content-type: application/json' \ -H 'x-API-key: ADYEN_API_KEY' \ -d '{ "merchantAccount": "YOUR_MERCHANT_ACCOUNT", "amount": { "value": 1000, "currency": "EUR" }, "returnUrl": "https://your-company.com/checkout?shopperOrder=12xy..", "reference": "YOUR_PAYMENT_REFERENCE", "mode": "hosted", "themeId": "AZ1234567", "countryCode": "NL", "expiresAt": "2023-05-18T10:15:30+01:00" }'
The /sessions response includes the URL (url
) for the Hosted Checkout page.
For example:
{ "id": "WNKH9MC2XJMLNK82", "merchantAccount": "TestMerchant", "amount": { "currency": "EUR", "value": 100 }, "returnUrl": "https://test-merchant….", "reference": "YOUR_REFERENCE", "countryCode": "NL", "expiresAt": "2023-15-05T19:31:22+01:00", "url": "https://eu.adyen.link/WNKH9MC2XJMLNK82" }
By default, the Hosted Checkout page expires (expiresAt
) 1 hour after it was created.
Redirect the shopper to the Hosted Checkout page
Redirect the shopper to the URL (url
) from the /sessions response. The shopper pays on the Hosted Checkout page.
Get the payment outcome
After finishes the payment flow, you can show the shopper the current payment status. Adyen sends a webhook with the outcome of the payment.
1. Show the result of the payment session
-
After the shopper makes the payment, they are redirected back to your website.
-
Get the
sessionId
andsessionResult
that is appended to the return URL from the Hosted Checkout page. Use it to get the outcome of the payment session.Return URL from Hosted Checkout pageExpand viewCopy link to code blockCopy codehttps://your-company.com/checkout?sessionId=WNKH9MC2XJMLNK82&sessionResult=QXhlbFN0b2x0ZW5iZXJnCg
-
Make a GET
/sessions/{id}?sessionResult={sessionResult}
request including thesessionId
andsessionResult
. For example:Get outcome of payment sessionExpand viewCopy link to code blockCopy codecurl -X GET https://checkout-test.adyen.com/checkout/v70/sessions/WNKH9MC2XJMLNK82?sessionResult=QXhlbFN0b2x0ZW5iZXJnCg
The response includes the current status (
status
) of the payment. For example:Response for outcome of payment sessionExpand viewCopy link to code blockCopy code{ "id": "CS12345678", "status": "completed" } Possible statuses:
status
Description completed The shopper completed the payment. paymentPending The shopper is in the process of making the payment. Applies to payment methods with an asynchronous flow, such as a voucher payment. canceled The shopper selected the Back button to return to your returnUrl
and cancel the payment.expired The session expired. The shopper can no longer use the Hosted Checkout page to make a payment.
The status
included in the response doesn't change. Do not make the request again to check for payment status updates. Instead, check webhooks or the Transactions list in your Customer Area.
Update your order management system
You get the outcome of each payment asynchronously, in an AUTHORISATION webhook. Use the merchantReference
from the webhook to match it to your order reference.
For a successful payment, the event contains success
: true.
{ "live": "false", "notificationItems":[ { "NotificationRequestItem":{ "eventCode":"AUTHORISATION", "merchantAccountCode":"YOUR_MERCHANT_ACCOUNT", "reason":"033899:1111:03/2030", "amount":{ "currency":"EUR", "value":2500 }, "operations":["CANCEL","CAPTURE","REFUND"], "success":"true", "paymentMethod":"mc", "additionalData":{ "expiryDate":"03/2030", "authCode":"033899", "cardBin":"411111", "cardSummary":"1111", "checkoutSessionId":"CSF46729982237A879" }, "merchantReference":"YOUR_REFERENCE", "pspReference":"NC6HT9CRT65ZGN82", "eventDate":"2021-09-13T14:10:22+02:00" } } ] }
For an unsuccessful payment, you get success
: false, and the reason
field has details about why the payment was unsuccessful.
{ "live": "false", "notificationItems":[ { "NotificationRequestItem":{ "eventCode":"AUTHORISATION", "merchantAccountCode":"YOUR_MERCHANT_ACCOUNT", "reason":"validation 101 Invalid card number", "amount":{ "currency":"EUR", "value":2500 }, "success":"false", "paymentMethod":"unknowncard", "additionalData":{ "expiryDate":"03/2030", "cardBin":"411111", "cardSummary":"1112", "checkoutSessionId":"861631540104159H" }, "merchantReference":"YOUR_REFERENCE", "pspReference":"KHQC5N7G84BLNK43", "eventDate":"2021-09-13T14:14:05+02:00" } } ] }
Payment errors and retries
If the payment encounters an error, the shopper can retry the payment on the Hosted Checkout page. You receive a webhook for each payment attempt. So, you can receive more than one webhook with the same sessionId
.
For a payment that encounters an error or fails on a redirect payment method's page, the shopper gets redirected to the Hosted Checkout page to retry the payment.
Expiration
The Hosted Checkout page expires after either:
- The
expiresAt
from the /sessions response. The default is 1 hour after it was created. - Our system determines that the shopper made too many payment attempts.
Test and go live
Before going live, use our list of test cards and other payment methods to test your integration. We recommend testing each payment method that you intend to offer to your shoppers.
You can check the test payments in your Customer Area, under Transactions > Payments.
When you are ready to go live, you need to:
- Apply for a live account.
- Configure your live account.
- Submit a request to add payment methods in your live Customer Area .
- Switch from test to our live endpoints.
See also
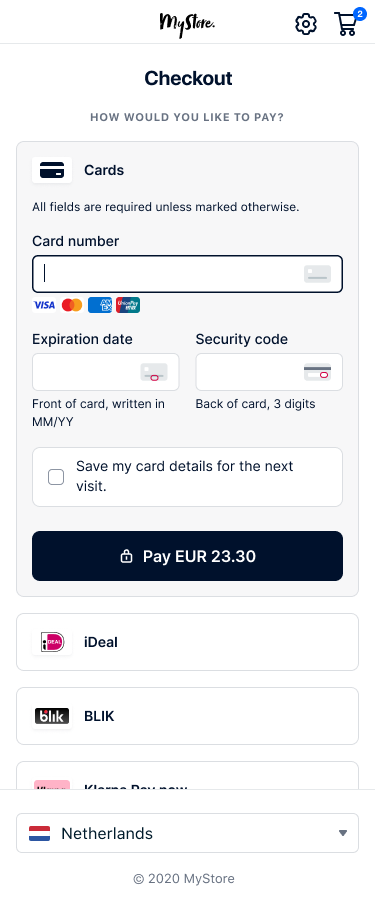
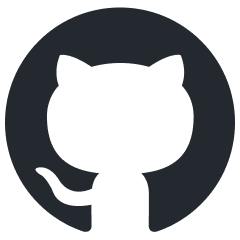
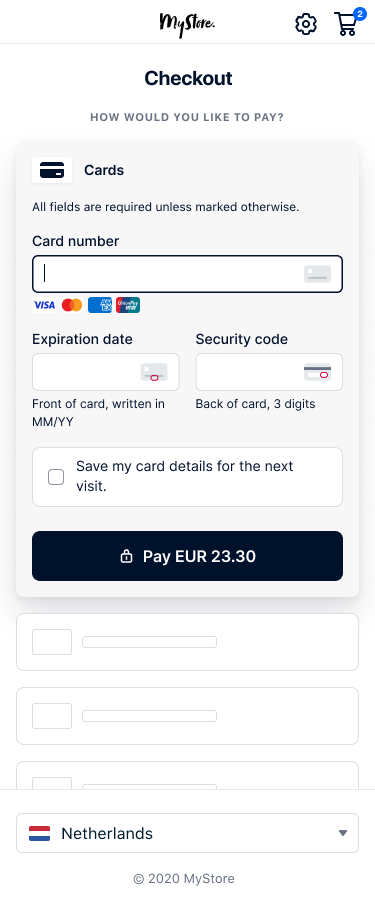
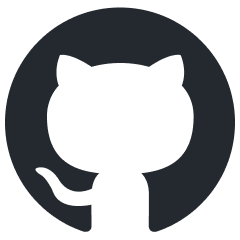
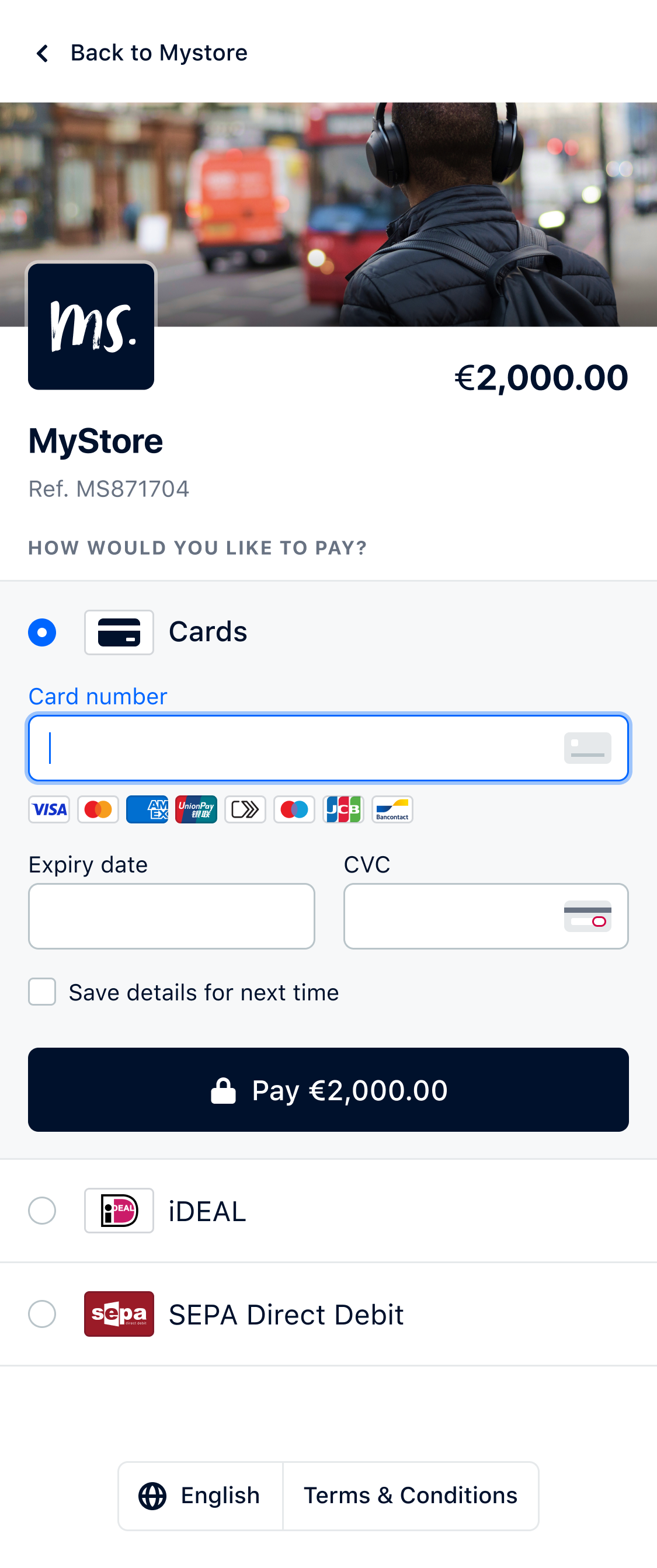
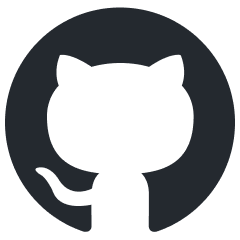
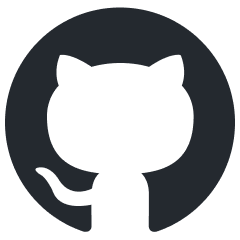
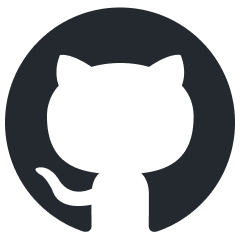
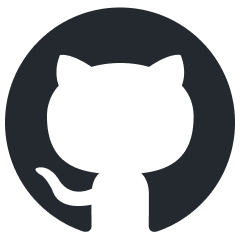
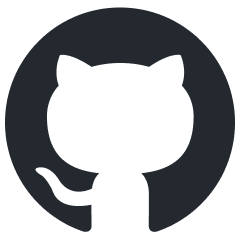
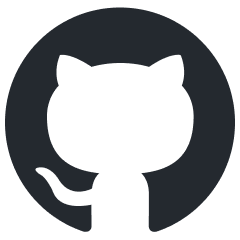
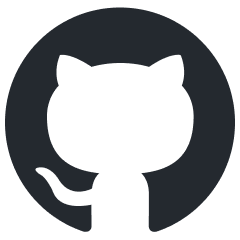